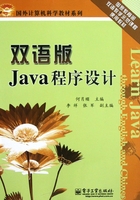
2.1 Concepts of OOP
As you know, all computer programs include two elements: code and data. Conceptually, a program can be organized based on code and data. That is, some programs are written around “what is happening” and others are written around “who is being affected”. These are the two examples that show how a program is constructed. The first way is called the process-oriented model(面向过程模式).
所有程序一般包括两个元素:代码和数据。其中,一些程序侧重处理的过程,一些程序侧重处理的对象。
This approach characterizes a program as a series of linear steps (that is, code). The process-oriented model can be viewed of as series of linear steps (that is, code). In the process-oriented model code is acted on data. C is paradigm for using this model. However problems appear as program grows larger and more complex.
To manage increasing complexity(复杂性), the second approach was invented. We called it called object-oriented programming(面向对象编程). This model is described as data controlling access to code. As you will know, by switching the controlling entity to data, you can achieve several organization benefits.
面向对象编程能够管理比较复杂的项目,这种编程模型使用数据来控制访问代码。
The following problem is that how to obtain object. The same types of entity have the same properties. So we can extract those same features from real-entities. This approach is called abstraction.
Abstraction: Abstraction is the presentation of simple concept (or object) to the external world. Abstraction concept is to describe a set of similar concrete entities. It focuses on the essential, inherent aspects of an entity and ignoring its accidental properties. The concept is usually used during analysis: deciding only with application-domain concepts, not making design and implementation decisions.
抽象是对外部世界简单概念(对象)的表达,是对同类实体集合的描述。它侧重于实体的本质、内在的方面,而忽略其偶然性。抽象概念通常在分析阶段使用,在设计阶段和实施阶段不使用。
Two popular abstractions: procedure abstraction and data abstraction. Procedure abstraction is to decompose problem to many simple sub-works. Data abstraction is to collect essential elements composing to a compound data. These two abstractions have the same objective: reusable and replaceable. Figure 2.1 shows an example of data abstraction to abstract doors as a data structure with essential properties.

Figure 2.1 Abstract of Door
Hierarchical classification is used to manage abstraction. This allows you to layer the semantics of complex systems, breaking them into more manageable pieces. From the outside, the car is a single object. Once inside, you see that the car consists of several subsystems: steering, brakes, sound system, seat belts, heating, cellular phone, and so on. In turn, each of these subsystems is made up of more specialized units. For instance, the sound system consists of a radio, a CD player, and /or tape player. The point is that you manage the complexity of the car (or any other complex system) through the use of hierarchical abstraction.
层次化分类用来管理抽象,允许将一个复杂的系统分层,将一个系统分解成一些可以管理的部分。
2.1.1 Class
A class is a construct that is used as a blueprint to create instances of itself. For example: There may be thousands of other bicycles in existence, all of the same make and model. Each bicycle was built from the same set of blueprints and therefore contains the same components. In object-oriented terms, we say that your bicycle is an instance of the class of objects known as bicycles. A class also is the blueprint from which individual objects are created.
类是一种被用来创建实例的模板。
2.1.2 Object
In everyday life, an object is anything that is identifiably a single material item. An object can be a car, a house, a book, a document, or a paycheck. For our purposes, we’re going to extend that concept a bit and think of an object as anything that is a single item that you might want to represent in a program. We’ll therefore also include living “objects”, such as a person, an employee, or a customer, as well as more abstract “objects”, such as a company, a database, or a country.
任何事物都可以看作对象,对象可以代表整个程序,也可以包括一些有生命的“对象”,如一个人,雇员,顾客,还包括一些比较抽象的“对象”,如一个企业,一个数据库或一个国家。
2.1.3 Encapsulation
Encapsulation is the mechanism that binds together code and the data it manipulates, and keeps both safe from outside interference and misuse.
Encapsulation allows an object to separate its interface from its implementation. The data and the implementation code for the object are hidden behind its interface. So encapsulation hides internal implementation details from users. The power of encapsulated code is that everyone knows how to access it and thus can use it regardless of the implementation details and without fear of unexpected side effects.
封装将对象的接口与实现分开,实现对数据和代码的隐藏,提高数据的安全性。
In Java the basis of encapsulation is the class. A class defines the structure and behavior (data and code) that will be shared by a set of objects. Each object of a given class contains the structure and behavior defined by the class, as if it were stamped out by a mold in the shape of the class. For this reason, objects are sometimes referred to as instance of class. Thus, a class is a logical construct; an object has physical reality.
When you create a class, you will specify the code and data that constitute that class. Collectively, these elements are called members of the class. Specifically the code that operates on that data is referred to as member variables or instance variables. The code that operates on that data is referred to as member methods or just methods. (If you are familiar with C/C++, it may help to know that what a Java programmer calls a method a C/C++ programmer calls a function.) In properly written Java programs, the methods define how the member variables can be used. This means that the behavior and interface of a class are defined by the methods that operate its instance data.
类由代码和数据组成,代码和数据是类的成员。类本身体现了封装特性。
2.1.4 Inheritance
In object-oriented programming (OOP), inheritance is a way to compartmentalize and reuse code by creating collections of attributes and behaviors called objects which can be based on previously created objects. In classical inheritance where objects are defined by classes, classes can inherit other classes. The new classes, known as subclasses (or derived classes), inherit attributes and behavior (i.e. previously coded algorithms) of the pre-existing classes, which are referred to as superclasses, ancestor classes or base classes. The inheritance relationships of classes give rise to a hierarchy. In prototype-based programming, objects can be defined directly from other objects without the need to define any classes, in which case this feature is called differential inheritance.The inheritance concept was invented in 1967 for Simula.
在面向对象编程(OOP)中,继承可以通过创造一系列属性和行为的方式来划分和重用代码。这些属性和方法依赖先前已经创建的对象,从而提高代码的重用率。
Without the use of hierarchies, each object would need to define all of its characteristics explicitly. However, by use of inheritance, an object need only define those qualities that make it unique within its class. It can inherit its general attributes from its parent. Thus, it is the inheritance mechanism that makes it possible for one object to be a specific instance of a more general case. Let’s take a closer look at this process.
Most people naturally view the world as made up of objects that are related to each other in hierarchical way, such as animals, mammals, and dogs. If you wanted to describe animals in an abstract way, you would say they have some attributes, such as size intelligence, and type of skeletal system. Animals also have certain behavioral aspects; they eat, breathe, and sleep. This description of attributes and behavior is the class definition for animals.
2.1.5 Polymorphism
Polymorphism (from the Greek, meaning “many forms”) is the ability to create a variable, a function, or an object that has more than one form. In Java, polymorphism is the ability of objects to react differently when presented with different information, known as parameters. In a functional programming language, it is the only way to complete two different tasks is to have two functions with different names.
多态性:发送消息给某个对象,让该对象自行决定响应何种行为。通过将子类对象引用赋值给超类对象引用变量,动态实现方法调用。