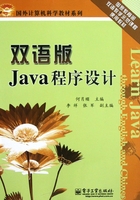
3.3 Casting
Because Java is a strongly typed language, it is sometimes necessary to perform a cast of a variable. Casting is the explicit(显式) or implicit(隐式) modification(修改) of a variable’s type. Remember before when I mentioned that once you declare a variable as a specific type, it has that type indefinitely? Well, casting allows us to view the data within a given variable as a different type than it was given. Casting is a rather large topic (especially when dealing with reference types). Instead of trying to tackle(解决) all of it, I’ll just hit on a few aspects relating to Java’s primitive data types.
类型转换允许将一个变量的类型改变为不同的类型。
In order to perform a cast, you place the type that you want to view the data as in parenthesis prior to the variable you want to cast, like this:
1: short x = 10; 2: int y = (int)x;
This code snippet(片段) causes the data in x, which is of type short, to be turned into an int and that value is placed into y. Please note, the variable x is not changed! The value within x is copied into a new variable (y), but as an int rather than a short.
There are two types of conversions that can be done to data, widening conversions and narrowing conversions. Widening conversions can be done implicitly (by the compiler, that is) and narrowing conversions must be done explicitly, by you, the programmer. The difference is simple. A widening conversion is when you take a variable and assign it to a variable of larger size. A narrowing conversion is when you take a variable and assign it to a variable of smaller size. Let’s look at some examples:
对数据来讲有两种转换方式:扩展转换和收缩转换。扩展转换是隐式的(通过编译器),而收缩转换必须显式声明。
3.3.1 Widening
1: byte x = 100; // Size = 1 byte 2: // In memory: x = 01100100 = 100 3: short y; // Size = 2 bytes 4: // In memory: y = 0000000000000000 = 0 5: y = x; // Legal 6: // In memory: y = 0000000001100100 = 100
3.3.2 Narrowing
1: short x = 1000; // Size = 2 bytes 2: // In memory: x = 0000001111101000 = 1000 3: byte y; // Size = 1 byte 4: // In memory: y =00000000 = 0 5: y = x; // Illegal 6: // Not enough space to copy all data 7: y = (byte)x; // Legal 8: // In memory: y =11101000 = 232
You can see from the first example that it is easy to copy the contents of a byte into a short because a short has enough space to accommodate whatever data was originally stored in the byte. In the second example, however, there isn’t enough space in a byte to accommodate what may be in the short. In order to get around that, you are required to explicitly cast, as seen in the seventh line. Notice, however, even though you are allowed to perform this cast, some data may be lost, as was the case in this example. This potential data loss is the reason for the required explicit cast.
字节型转换为短整型只是简单地将内容复制,因为短整型提供了足够大的空间来存储原存储在字节型中的任何数。如果没有提供足够的空间来存储短整型,为了实现这种转换就必须显式地进行转换。