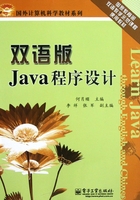
4.1 Arithmetic Operators
Arithmetic operators are used in mathematical expression(数学表达式)in the same way that they are used in algebra(代数学). The Table 4.1 lists the arithmetic operators:
Table 4.1 Arithmetic Operators
The operands of the arithmetic operators must be of numeric type. You cannot use them on boolean types, but you can use them on char types, since the char type in Java is, essentially, a subset of int.
class example1 { public static void main(String args[]) { System.out.println("Integer Arithmetic"); int a = 1 + 1; System.out.println("a="+a); } }
when you run this program you will see the following output:
Integer Arithmetic
a = 2
4.1.1 The Modulus Operators
The modulus operator, %, return the remainder of a division operation. It can be applied to floating-point types as well as integer types. The following program demonstrates.
求余运算符%,返回除法操作的余数。它可以应用于浮点型,也可以应用于整型。下面将给出示例。
class example2 { public static void main(String args[]) { int x = 42; double y = 42.3; System.out.println("x mod 10 = " + x % 10); System.out.println("y mod 10 = " + y % 10); } }
when you run this program you will get following output:
x mod 10 = 2
y mod 10 = 2.299999999999997
4.1.2 Arithmetic Assignment Operators
Java provides special operators that can be used to combine(结合) an arithmetic operation with an assignment. As you probably know, statements like the following are quite common in programming.
a=a+4;
In Java, you can write this statement as shown here :
a+=4;
This version uses the += assignment operator. Both statements performs the same action: they increase the value of a by 4;here are some more examples.
a=a%2; can be written as a%=2; b=b*3; can be written as b*=3; c=c/5; can be written as c/=5; d=d-7; can be written as d-=7;
4.1.3 Increment and Decrement
The ++ and the - are Java’s increment and decrement operators. The increment operator increases its operand by one. The decrement operator decreases its operand by one.
For example, this statement x=x+1; can be rewritten like this by use of increment operator x++;
Similarly, this statement x=x-1; is equivalent to x--;
These operators are unique in that they can appear both in postfix form, where they follow the operand as just shown, and prefix form, where they precede the operand.
当这些运算符跟在操作数的后面时,以后缀形式出现;当这些运算符放在操作数的前面时,以前缀形式出现。
class example3 { public static void main(String args[]) { int a = 23; int b = 5; System.out.println("a & b : " + a + " " + b); a += 30; b *= 5; System.out.println("after arithmetic assignment a & b: "+a+" "+b); a++; b--; System.out.println("after increment & decrement a & b: "+a+" "+b); } }
when you run this program you will get following output:
a & b : 23 5
after arithmetic assignment a & b : 53 25
after increment & decrement a & b : 54 24