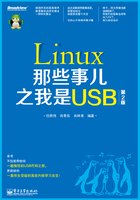
32.驱动的生命线(二)
Core配置设备使用的是message.c里的usb_set_configuration函数。
1430 int usb_set_configuration(struct usb_device *dev, int configuration) 1431 { 1432 int i, ret; 1433 struct usb_host_config *cp = NULL; 1434 struct usb_interface **new_interfaces = NULL; 1435 int n, nintf; 1436 1437 if (configuration == -1) 1438 configuration = 0; 1439 else { 1440 for (i = 0; i < dev->descriptor.bNumConfigurations; i++) { 1441 if (dev->config[i].desc.bConfigurationValue == 1442 configuration) { 1443 cp = &dev->config[i]; 1444 break; 1445 } 1446 } 1447 } 1448 if ((!cp && configuration != 0)) 1449 return -EINVAL; 1450 1451 /* The USB spec says configuration 0 means unconfigured. 1452 * But if a device includes a configuration numbered 0, 1453 * we will accept it as a correctly configured state. 1454 * Use -1 if you really want to unconfigure the device. 1455 */ 1456 if (cp && configuration == 0) 1457 dev_warn(&dev->dev, "config 0 descriptor??\n"); 1458 1459 /* Allocate memory for new interfaces before doing anything else, 1460 * so that if we run out then nothing will have changed. */ 1461 n = nintf = 0; 1462 if (cp) { 1463 nintf = cp->desc.bNumInterfaces; 1464 new_interfaces = kmalloc(nintf * sizeof(*new_interfaces), 1465 GFP_KERNEL); 1466 if (!new_interfaces) { 1467 dev_err(&dev->dev, "Out of memory"); 1468 return -ENOMEM; 1469 } 1470 1471 for (; n < nintf; ++n) { 1472 new_interfaces[n] = kzalloc( 1473 sizeof(struct usb_interface), 1474 GFP_KERNEL); 1475 if (!new_interfaces[n]) { 1476 dev_err(&dev->dev, "Out of memory"); 1477 ret = -ENOMEM; 1478 free_interfaces: 1479 while (--n >= 0) 1480 kfree(new_interfaces[n]); 1481 kfree(new_interfaces); 1482 return ret; 1483 } 1484 } 1485 1486 i = dev->bus_mA - cp->desc.bMaxPower * 2; 1487 if (i < 0) 1488 dev_warn(&dev->dev, "new config #%d exceeds power " 1489 "limit by %dmA\n", 1490 configuration, -i); 1491 } 1492 1493 /* Wake up the device so we can send it the Set-Config request */ 1494 ret = usb_autoresume_device(dev); 1495 if (ret) 1496 goto free_interfaces; 1497 1498 /* if it's already configured, clear out old state first. 1499 * getting rid of old interfaces means unbinding their drivers. 1500 */ 1501 if (dev->state != USB_STATE_ADDRESS) 1502 usb_disable_device (dev, 1); // Skip ep0 1503 1504 if ((ret = usb_control_ msg(dev, usb_sndctrlpipe(dev, 0), 1505 USB_REQ_SET_CONFIGURATION, 0, configuration, 0, 1506 NULL, 0, USB_CTRL_SET_TIMEOUT)) < 0) { 1507 1508 /* All the old state is gone, so what else can we do? 1509 * The device is probably useless now anyway. 1510 */ 1511 cp = NULL; 1512 } 1513 1514 dev->actconfig = cp; 1515 if (!cp) { 1516 usb_set_device_state(dev, USB_STATE_ADDRESS); 1517 usb_autosuspend_device(dev); 1518 goto free_interfaces; 1519 } 1520 usb_set_device_state(dev, USB_STATE_CONFIGURED); 1521 1522 /* Initialize the new interface structures and the 1523 * hc/hcd/usbcore interface/endpoint state. 1524 */ 1525 for (i = 0; i < nintf; ++i) { 1526 struct usb_interface_cache *intfc; 1527 struct usb_interface *intf; 1528 struct usb_host_interface *alt; 1529 1530 cp->interface[i] = intf = new_interfaces[i]; 1531 intfc = cp->intf_cache[i]; 1532 intf->altsetting = intfc->altsetting; 1533 intf->num_altsetting = intfc->num_altsetting; 1534 kref_get(&intfc->ref); 1535 1536 alt = usb_altnum_to_altsetting(intf, 0); 1537 1538 /* No altsetting 0? We'll assume the first altsetting. 1539 * We could use a GetInterface call, but if a device is 1540 * so non-compliant that it doesn't have altsetting 0 1541 * then I wouldn't trust its reply anyway. 1542 */ 1543 if (!alt) 1544 alt = &intf->altsetting[0]; 1545 1546 intf->cur_altsetting = alt; 1547 usb_enable_interface(dev, intf); 1548 intf->dev.parent = &dev->dev; 1549 intf->dev.driver = NULL; 1550 intf->dev.bus = &usb_bus_type; 1551 intf->dev.type = &usb_if_device_type; 1552 intf->dev.dma_mask = dev->dev.dma_mask; 1553 device_initialize (&intf->dev); 1554 mark_quiesced(intf); 1555 sprintf (&intf->dev.bus_id[0], "%d-%s:%d.%d", 1556 dev->bus->busnum, dev->devpath, 1557 configuration, alt->desc.bInterfaceNumber); 1558 } 1559 kfree(new_interfaces); 1560 1561 if (cp->string == NULL) 1562 cp->string = usb_cache_string(dev, cp->desc.iConfiguration); 1563 1564 /* Now that all the interfaces are set up, register them 1565 * to trigger binding of drivers to interfaces. probe() 1566 * routines may install different altsettings and may 1567 * claim() any interfaces not yet bound. Many class drivers 1568 * need that: CDC, audio, video, etc. 1569 */ 1570 for (i = 0; i < nintf; ++i) { 1571 struct usb_interface *intf = cp->interface[i]; 1572 1573 dev_dbg (&dev->dev, 1574 "adding %s (config #%d, interface %d)\n", 1575 intf->dev.bus_id, configuration, 1576 intf->cur_altsetting->desc.bInterfaceNumber); 1577 ret = device_add (&intf->dev); 1578 if (ret != 0) { 1579 dev_err(&dev->dev, "device_add(%s) --> %d\n", 1580 intf->dev.bus_id, ret); 1581 continue; 1582 } 1583 usb_create_sysfs_intf_files (intf); 1584 } 1585 1586 usb_autosuspend_device(dev); 1587 return 0; 1588 }
这个函数可以分成三个部分,从1432行到1491行的这几十行是准备阶段,做常规检查,申请内存。1498行到1520行这部分可是重头戏,就是在这里设备从Address发展到了Configured。1522行到1584行这个阶段也挺重要的,主要充实设备的每个接口并提交给设备模型,为它们寻找命中注定的接口驱动,过了这个阶段,usb_generic_driver也就彻底从设备那儿得到满足了,generic_probe的历史使命也就完成了。
先看第一阶段,1437行,configuration是从前面的choose_configuration()那里返回来的值,找到合适的配置,就返回那个配置的bConfigurationValue值,没有找到合适的配置,就返回-1,所以这里的configuration值就可能有两种情况,或者为-1,或者为配置的bConfigurationValue值。
当configuration为-1时,这里为什么又要把它改为0呢?要知道configuration这个值是要在后面的高潮阶段里发送SET_CONFIGURATION请求时用的,关于SET_CONFIGURATION请求,spec里说,这个值必须为0或者与配置描述符的bConfigurationValue一致。如果为0,则设备收到SET_CONFIGURATION请求后,仍然会待在Address状态。这里当configuration为-1也就是没有发现满意的配置时,设备不能进入Configured,所以要把configuration的值改为0,以便满足SET_CONFIGURATION请求的要求。
那接下来的问题就出来了,在没有找到合适配置时直接给configuration这个参数设置为0,也就是让choose_configuration()返回0不就得了,干吗还这么麻烦先返回个-1再把它改成0?有些设备就是有拿0当配置bConfigurationValue值的毛病,你又不能不让它用。想让设备回到Address状态时,usb_set_configuration()就不传递0了,传递一个-1,然后进行处理。如果configuration值为0或大于0的值,就从设备struct usb_device结构体的config数组里将相应配置的描述信息,也就是struct usb_host_config结构体给取出来。
1448行,如果没有拿到配置的内容,configuration值就必须为0了,让设备待在Address那里别动。这也很好理解,配置的内容都找不到了,还配置什么呢?当然,如果拿到了配置的内容,但同时configuration为0,这就是对应了上面说的那种有毛病的设备的情况,就提出警告出现不正常现象了。
1461行,过了这个if函数,第一阶段就告结束了。如果配置是实实在在存在的,就为它使用的接口都准备一个struct usb_interface结构体。new_interfaces是开头儿就定义好的一个struct usb_interface结构体指针数组,数组的每一项都指向了一个struct usb_interface结构体,所以这里申请内存也要分两步走,先申请指针数组的,再申请每一项的。