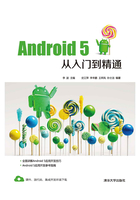
4.8 Toast和Notification
Toast和Notification是Android系统为用户提供的轻量级的信息提醒机制。这种方式不会打断用户当前的操作,也不会获取到焦点,非常方便。
本节我们通过实例学习Toast和Notification的使用方法。
4.8.1 Toast
创建工程NotificationDemo,并实现如图4.44布局。

图4.44 工程布局
main.xml代码如下:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical"> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Toast和Notification演示" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Toast" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Notification" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="CancelNotification" /> </LinearLayout>
在NotificationDemoActivity中为每个按钮添加事件响应。单击Toast按钮,运行效果如图4.45所示。

图4.45 单击Toast按钮的效果
相关代码如下:
Button toastBtn=(Button)this.findViewById(R.id.button1); toastBtn.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v){ // TODO Auto-generated method stub Toast.makeText(NotificationDemoActivity.this, "这是一个Toast演示!", Toast.LENGTH_LONG).show(); } });
Toast用于向用户显示小信息量的提示,它不会中断应用程序进程,不会对用户操作造成任何干扰,也不能与用户交互,在信息显示后会自动消失。此处使用Toast.makeText(Context context, CharSequence text, int duration)方法来创建一个Toast。其中context指显示Toast的上下文,text指Toast中显示的文字内容,duration指Toast显示延续的时间,该时间可以直接指定,也可以使用Toast提供LENGTH_LONG和LENGTH_SHORT常量。Toast.show()方法可以将Toast对象显示出来。Toast默认情况下显示在屏幕的下方,可以通过Toast.setGravity()方法设置Toast的显示位置。例如,如下代码:
Toast toast=Toast.makeText(NotificationDemoActivity.this, "这是一个位于中间位置的Toast", Toast.LENGTH_LONG); toast.setGravity(Gravity.CENTER, 0, 0); toast.show();
显示效果如图4.46所示。

图4.46 显示效果
4.8.2 Notification
Notification可以在手机屏幕顶部的状态栏显示一个带图标的通知,同时播放声音或者使手机震动。Notification可以扩展以显示详细信息,单击该Notification还可以跳转到特定的Activity。
单击Notification按钮,运行效果如图4.47所示,在视图的状态栏出现Notification提示。按住Notification并下拉,可将Notification内容进行扩展,效果如图4.48所示。单击图标处,应用程序跳转到NoteActivity视图,运行效果如图4.49所示。单击“返回”按钮,返回到NotificationDemoActivity视图。

图4.47 单击Notification 按钮的效果

图4.48 下拉Notification 的效果

图4.49 单击图标的效果
相关代码如下:
Button notifyBtn=(Button)this.findViewById(R.id.button2); notifyBtn.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v){ // TODO Auto-generated method stub context=getApplicationContext(); String ns=Context.NOTIFICATION_SERVICE; mNotificationManager=(NotificationManager)getSystemService(ns); int icon=R.drawable.icon01; CharSequence tickerText="这是一个Notification!"; long when=System.currentTimeMillis(); Notification.Builder builder=new Notification.Builder(context); builder.setSmallIcon(icon); builder.setTicker(tickerText); builder.setWhen(when); notification=builder.getNotification(); CharSequence contentTitle="My notification"; CharSequence contentText="单击这个notification,可以跳转到NoteActivity."; Intent notificationIntent=new Intent(context, NoteActivity.class); PendingIntent contentIntent=PendingIntent.getActivity(context, 0, notificationIntent, 0); notification.setLatestEventInfo(context, contentTitle, contentText, contentIntent); notification.defaults=notification.DEFAULT_SOUND; mNotificationManager.notify(NOTIFICATION_ID, notification); } });
Notification.Builder是Android API Level 11以上版本提供的Notification的创建类,可以方便地创建Notification并设置各种属性。此处创建了一个Notification,并指定了显示内容和图标。Notification.setLatestEventInfo()方法设定了当用户扩展Notification时显示的样式,并通过PendingIntent对象指定了当用户单击扩展的Notification时应用程序如何跳转,此处跳转至NoteActivity。NotificationManager.notify(int id,Notification notification)方法为Notification对象指定一个id值,并将该Notification对象显示到状态栏上。NotificationManager.cancel(int id)方法会将id指向的Notification对象取消掉。
NoteActivity.java代码如下:
package introduction.android.notificationDemo; import android.app.Activity;import android.content.Intent;import android.os.Bundle;import android.view.View;import android.widget.Button; public class NoteActivity extends Activity { private Button btn; public void onCreate(Bundle savedInstanceState){ super.onCreate(savedInstanceState); setContentView(R.layout.other); btn=(Button)this.findViewById(R.id.button1); btn.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v){ // TODO Auto-generated method stub Intent intent=new Intent(NoteActivity.this,NotificationDemoActivity.class); startActivity(intent); } }); } }
NoteActivity所使用的布局文件other.xml代码如下:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="NoteActivity" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="返回" /> </LinearLayout>