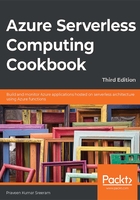
Sending an email notification dynamically to the end user
In the previous recipe, we hard-coded most of the attributes related to sending an email to an administrator as there was just one administrator. In this recipe, we will modify the previous recipe to send a Thank you for registration email to the users themselves.
Getting ready
Make sure that the following steps are configured properly:
- The SendGrid account is created and an API key is generated in the SendGrid portal.
- An App settings configuration is created in the configuration of the function app.
- The App settings key is configured in the SendGrid output (message) bindings.
How to do it…
In this recipe, we will update the code in the run.csx file of the following Azure functions:
- RegisterUser
- SendNotifications
Accepting the new email parameter in the RegisterUser function
Let's make changes to the RegisterUser function to accept the email parameter by performing the following steps:
- Navigate to the RegisterUser function, in the run.csx file, and add a new string variable that accepts a new input parameter, named email, from the request object, as follows. Also, note that we are serializing the UserProfile object and storing the JSON content to the queue message:
string firstname=null,lastname = null, email = null;
...
...
email = inputJson.email;
...
...
UserProfile objUserProfile = new UserProfile(firstname, lastname, string profilePicUrl,email);
...
...
await NotificationQueueItem.AddAsync(JsonConvert.SerializeObject(objUserProfile))
;
- Update the following code to the UserProfile class and click on the Save button to save the changes:
public class UserProfile : TableEntity
{
public UserProfile (string firstname, string lastname, string profilePicUrl, string email)
{
....
....
this.ProfilePicUrl = profilePicUrl;
this.Email = email;
}
....
....
public string ProfilePicUrl {get; set;}
public string Email {get; set;}
}
Let's now move on to retrieve the user profile information.
Retrieving the UserProfile information in the SendNotifications trigger
In this section, we will perform the following steps to retrieve the user information:
- Navigate to the SendNotifications function, in the run.csx file, and add the NewtonSoft.Json reference and also the namespace.
- The queue trigger will receive the input in the form of a JSON string. We will use the JsonConvert.Deserializeobject method to convert the string into a dynamic object so that we can retrieve the individual properties. Replace the existing code with the following code where we are dynamically populating the properties of SendGridMessage from the code:
#r "SendGrid"
#r "Newtonsoft.Json" using System;
using SendGrid.Helpers.Mail;
using Newtonsoft.Json;
public static void Run(string myQueueItem,out SendGridMessage message, ILogger log)
{
log.LogInformation($"C# Queue trigger function processed:
{myQueueItem}");
dynamic inputJson = JsonConvert.DeserializeObject(myQueueItem); string FirstName=null, LastName=null, Email = null; FirstName=inputJson.FirstName;
LastName=inputJson.LastName; Email=inputJson.Email;
log.LogInformation($"Email{inputJson.Email}, {inputJson.FirstName
+ " " + inputJson.LastName}");
message = new SendGridMessage();
message.SetSubject("New User got registered successfully."); message.SetFrom("donotreply@example.com"); message.AddTo(Email,FirstName + " " + LastName);
message.AddContent("text/html", "Thank you " + FirstName + " " + LastName +" so much for getting registered to our site.");
}
- After making all of the aforementioned highlighted changes to the SendNotifications function, click Save. In order to test this, you need to execute the RegisterUser function. Let's run a test by adding a new input field email to the test request payload of the RegisterUser function, shown as follows:
{
"firstname": "Praveen",
"lastname": "Sreeram",
"email":"example@gmail.com",
"ProfilePicUrl":"A valid url here"
}
- This is the screenshot of the email that I have received:
Figure 2.16: Email notification of successful registration
How it works...
We have updated the code of the RegisterUser function to accept another new parameter, named email.
The function accepts the email parameter and sends the email to the end user using the SendGrid API. We have also configured all the other parameters, such as the From address, subject, and body (content) in the code so that it can be customized dynamically based on the requirements.
We can also clear the fields in the SendGrid output bindings, as shown in Figure 2.17:

Figure 2.17: Clearing the fields in the SendGrid output bindings
Note
The values specified in the code will take precedence over the values specified in the preceding step.
There's more...
You can also add HTML content in the body to make your email look more attractive. The following is a simple example where I have just applied a bold (<b>) tag to the name of the end user:
message.AddContent("text/html", "Thank you <b>" + FirstName + "</b><b> " + LastName +" </b>so much for getting registered to our site.");
Figure 2.18 shows the email, with my name in bold:

Figure 2.18: Customizing the email notification
In this recipe, you have learned how to send an email notification dynamically to the end user. Let's now move on to the next recipe.