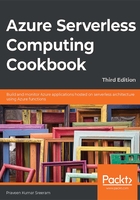
Sending an SMS notification to the end user using the Twilio service
In most of the previous recipes of this chapter, we have worked with SendGrid triggers to send emails in different scenarios. In this recipe, you will learn how to send notifications via text messages, using one of the leading cloud communication platforms, named Twilio.
Note
Twilio is a cloud communication platform-as-a-service platform. Twilio allows software developers to programmatically make and receive phone calls, send and receive text messages, and perform other communication functions using its web service APIs. Learn more about Twilio at https://www.twilio.com/.
Getting ready
In order to use the Twilio SMS output (objsmsmessage) binding, you need to do the following:
- Create a trial Twilio account at https://www.twilio.com/try-twilio.
- Following the successful creation of the account, grab the ACCOUNT SID and AUTH TOKEN from the Twilio Dashboard and save it for future reference, as shown in Figure 2.23. You need to create two App settings in the Configuration blade of the function app for both of these settings:
Figure 2.23: Twilio dashboard
- In order to start sending messages, you need to create an active number within Twilio, which will be used as the From number that you will use to send the SMS. You can create and manage numbers in the Phone Numbers Dashboard. Navigate to https://www.twilio.com/console/phone-numbers/incoming and click on the Get Started button.
On the Get Started with Phone Numbers page, click on Get your first Twilio phone number, as shown in Figure 2.24:
Figure 2.24: Activating your number using Twilio
- Once you get your number, it will be listed as follows:
Figure 2.25: Displaying the activated number
- The final step is to verify a number to which you would like to send an SMS. Click on the + icon, as shown in Figure 2.26, provide your number, and then click on the Call Me button:
Figure 2.26: Verifying a phone number
- You can have only one number in your trial account, which can be verified on Twilio's verified page: https://www.twilio.com/console/phone-numbers/verified. Figure 2.27 shows the list of verified numbers:
Figure 2.27: Verified caller IDs
How to do it...
Perform the following steps:
- Navigate to the Application settings blade of the function app and add two keys for storing TwilioAccountSID and TwilioAuthToken, as shown in Figure 2.28:
Figure 2.28: Adding two keys for storing TwilioAccountSID and TwilioAuthToken
- Go to the Integrate tab of the SendNotifications function, click on New Output, and choose Twilio SMS.
- Click on Select and provide the following values to the Twilio SMS output bindings. Please install the extensions of Twilio. To manually install the extensions, refer to the https://docs.microsoft.com/azure/azure-functions/install-update-binding-extensions-manual article. The From number is the one that is generated in the Twilio portal, which we discussed in the Getting ready section of this recipe:
Figure 2.29: Twilio SMS output blade
- Navigate to the code editor and add the following lines of code. In the following code, I have hard-coded the To number. However, in real-world scenarios, you would dynamically receive the end user's mobile number and send the SMS via code:
...
...
#r "Twilio"
#r "Microsoft.Azure.WebJobs.Extensions.Twilio"
...
...
using Microsoft.Azure.WebJobs.Extensions.Twilio; using Twilio.Rest.Api.V2010.Account;
using Twilio.Types;
public static void Run(string myQueueItem,
out SendGridMessage message, IBinder binder,
out CreateMessageOptions objsmsmessage,
ILogger log)
...
...
...
message.AddAttachment(FirstName +"_"+LastName+".log", System.Convert.ToBase64String(System.Text.Encoding.UTF8.GetBytes(emailContent)),
"text/plain",
"attachment",
"Logs"
);
objsmsmessage = new CreateMessageOptions(new PhoneNumber("+91 98492*****"));
objsmsmessage.Body = "Hello.. Thank you for getting registered.";
}
- Now, do a test run of the RegisterUser function using the same request payload.
- Figure 2.30 shows the SMS that I have received:
Figure 2.30: SMS received from the Twilio account
How it works...
We have created a new Twilio account and copied the account ID and app key to the App settings of the Azure Function app. The account ID and app key will be used by the function app runtime in order to connect to the Twilio API to send the SMS.
For the sake of simplicity, I have hard-coded the phone number in the output bindings. However, in real-world applications, you would send the SMS to the phone number provided by the end users.
Watch the following video to view a working implementation: https://www.youtube.com/watch?v=ndxQXnoDIj8.