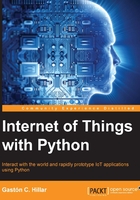
Improving our object-oriented code to provide new features
Now that we have our counter working with the LEDs connected to the board, we want to add new features. We want to be able to easily transform a number between 1 and 9 into its representation in LEDs connected to the board.
The following lines show the code for the new NumberInLeds
class. The code file for the sample is iot_python_chapter_03_05.py
.
class NumberInLeds: def __init__(self): self.leds = [] for i in range(1, 10): led = Led(i) self.leds.append(led) def print_number(self, number): print("==== Turning on {0} LEDs ====".format(number)) for j in range(0, number): self.leds[j].turn_on() for k in range(number, 9): self.leds[k].turn_off()
The constructor, that is, the __init__
method, declares an empty list attribute named leds
(self.leds
). Then, a for
loop creates nine instances of the Led
class and each of them represent an LED connected to a GPIO pin on the board. We pass i
as an argument for the pin
parameter. Then, we call the append
method for the self.leds
list to add the Led
instance (led
) to the self.leds
list. Our for
loop will start with i
equal to 1 and its last iteration will be with i
equal to 9.
The class defines a print_number
method that requires the number that we want to represent with LEDs turned on in the number
argument. The method uses a for
loop with j
as its variable to turn on the necessary LEDs by accesing the appropriate members of the self.leds
list and calling the turn_on
method. Then, the method uses another for
loop with k
as its variable to turn off the remaining LEDs by accesing the appropriate members of the self.leds
list and calling the turn_off
method. This way, the method makes sure that only the LEDs that have to be turned on are really turned on and the rest of them are turned off.
Now, we can write code that uses the new NumberInLeds
class to count from 0 to 9 with the LEDs. In this case, we start with 0 because the new class is able to turn off all the LEDs that shouldn't be turned on to represent a specific number. The code file for the sample is iot_python_chapter_03_05.py
.
if __name__ == "__main__": print ("Mraa library version: {0}".format(mraa.getVersion())) print ("Mraa detected platform name: {0}".format(mraa.getPlatformName())) number_in_leds = NumberInLeds() # Count from 0 to 9 for i in range(0, 10): number_in_leds.print_number(i) time.sleep(3)
The code is very easy to understand, we just create an instance of the NumberInLeds
class, named number_in_leds
, and then we call its print_number
method with i
as its argument within the for
loop.
Tip
We took advantage of Python's object-oriented features to create classes that represent the LEDs and the generation of numbers with LEDs. This way, we wrote higher level code that is easier to understand because we don't just read code that writes 0s and 1s to specific pin numbers, we can read code that prints numbers in LEDs, turns on and turns off LEDs.