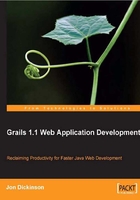
Create the domain classes
Grails uses the Model View Controller (MVC) pattern for web development. We will work on our model first. To allow the application to manage users, we will need a User
class and a Role
class. These must be created as domain classes in Grails. Domain classes constitute the domain model for the application. They are mapped directly to database tables by the Grails framework and form the central structure of an application. You can create new domain classes by using the supplied Grails command line tool.
Go to your console and enter:
>grails create-domain-class app.User
The output of this command should look like this:
Welcome to Grails 1.1 - http://grails.org/ Licensed under Apache Standard License 2.0 Grails home is set to: /tools/grails-1.1 Base Directory: /workspace/books/effective-grails/code-1.1/chapter02/teamwork Running script /tools/grails-1.1/scripts/CreateDomainClass.groovy Environment set to development [mkdir] Created dir: /workspace/books/effective-grails/code-1.1/chapter02/teamwork/grails-app/domain/app Created DomainClass for User [mkdir] Created dir: /workspace/books/effective-grails/code-1.1/chapter02/teamwork/test/unit/app Created Tests for User
Now, enter:
>grails create-domain-class app.Role
You should see from the messages in the console that both the User
and Role
classes have been created, along with a test class for each of them. The new classes will be created in the app
package.
Note
Always put your Grails classes in a package. Otherwise, you may have compilation problems if you implement utility classes in Java code that use your application classes. The Java compiler, as of JDK 1.4, does not allow classes from the unnamed or default
package to be imported into classes under a package (http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=4361575).
Create the User domain class
Go to the grails-app/domain/app
folder and open up the User.groovy
class. The vanilla class will be a simple empty Groovy class:
package app class User { static constraints = { } }
Add the following properties to the User
class:
- The username of the user, for authentication.
- The title of the user.
- The first name of the user.
- The last name of the user.
- The password the user must enter to be authenticated.
- The date the user was created.
- The date the user was last updated.
package app
class User {
String username String title String firstName String lastName String password Date dateCreated Date lastModified
static constraints = {
}
}
The given code looks a lot like Java code (there is a package and class definition and the properties have been declared as Java objects). However, there are a few issues that would stop this class compiling as Java code, namely:
- There are no semicolons marking the end of a statement
- The
Date
class is not imported - The class definition is missing the
public
keyword
This is all fine because it is not a Java class; it is a Groovy class. It is a reassuring fact that there is very little difference syntactically between Java and Groovy code. We will cover Groovy in more depth in Chapter 4.