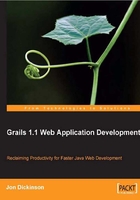
Create a home page
Given that the application only allows people to create messages at the moment, you might as well just make the home page show all the messages that have been created. To get the home page up and running, you will need to do the following:
- Create a new controller for the home page to retrieve all messages
- Create a new GSP to display the messages
- Modify the main layout to use the right stylesheet for your application
HomeController
The HomeController
will retrieve the information to be displayed on the home page. This needs one action, to retrieve the messages from the database in the descending order of date. Create a file called HomeController.groovy
in the grails-app/controllers/app
directory and add the following code:
package app class HomeController { def index = { def messages = Message.list( sort: 'lastUpdated', order: 'desc' ) return [messages: messages] } }
The index
action is the default action for controllers. This allows users to access the action without having to specify the action name on the URL. Both the following URLs would execute this action:
http://localhost:8080/teamwork/home/index
http://localhost:8080/teamwork/home
You can query the database for instances of Message
using the list
method that Grails adds on to all the domain classes. By default, the list
method will return all the objects of the class the method is called on. In your example, you specify that the lastUpdated
property will be used for sorting, and that the sort order will be descending.
The following are valid parameters for the list
method:

Apart from the current usage, some examples will illustrate these parameters:
Message.list():
This will retrieve all instances of theMessage
from the database.Message.list( sort: 'lastUpdated', order: 'desc', max: 20 ):
This will retrieve the twenty most recently updated messages.Message.list( sort: 'lastUpdated', order: 'desc', offset: 20, max: 20 ):
This will retrieve the twenty next most recently updated messages.Message.list( fetch: [ user: 'eager' ] ):
This will retrieve all instances of message and populate theuser
property. When the default fetch strategy (lazy loading) isused
, theuser
property on themessage
objects will not be populated. Instead, Hibernate will wait until theuser property
is accessed, and then load the related user data from the database. Currently, there is nouser
property onMessage
this will change in Chapter 5, where you will see why and when to specify the fetch strategy.
Home page view
Now to work on the Home Page view, you must create a GSP file called index.gsp
in the grails-app/views/home
directory and replace its contents with the following:
<%@ page contentType="text/html;charset=UTF-8" %>
<html>
<head>
<meta http-equiv="Content-Type"
content="text/html; charset=UTF-8"/>
<meta name="layout" content="main"/>
<title>Home</title>
</head>
<body>
<h2>Messages</h2>
<g:if test="${flash.toUser}"> <div id="userMessage" class="info"> ${flash.toUser} </div> </g:if> <g:each in="${messages}" var="message"> <div class="amessage"> <div class="messagetitle"> ${message.title} </div> <div class="messagebody"> ${message.detail} </div> </div> </g:each>
</body>
</html>
The aim of the home page is to show a list of all the messages that have been posted in the descending order of date.
You should recognize the first block of code on the home page. It will display a user message that has been added to the flash scope. This has been included because users will be sent to the home page once they have created a message in future.
The second highlighted section iterates through the list of messages that have been retrieved from the database by the index
action in HomeController
. It then prints out the title and the detail of the message. The Grails each
tag allows you to specify the collection to be iterated over using the in
attribute. You then declare the variable name to be used for each item using the var
attribute.
Before viewing the home page to check that everything is working, add some messages to the BootStrap
class, so that you have the test data available whenever you restart the application:
import app.Role
import app.Message
class BootStrap {
def init = { servletContext ->
def user = new Role(name: 'User').save()
def admin = new Role(name: 'Administrator').save()
new Message( title:'The Knights Who Say Nee',
detail:'They are after a shrubbery.' ).save()
new Message( title:'The Black Knight',
detail:"Just a flesh wound." ).save()
new Message( title:'air speed velocity of a swallow',
detail:"African or European?" ).save()
}
def destroy = {
}
}
You should now be able to go to the URL for the home page ( http://localhost:8080/teamwork/home
) and see the list of messages that have been posted so far, with the most recent message at the top.
