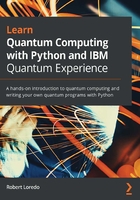
Understanding entanglement
Entanglement is probably one of the most interesting of the three quantum computing principles. This is mainly because it still baffles physicists and scientists to this day, with many taking different philosophical sides on the discussion. I won't bore you with the details, but I will definitely provide you with enough information for you to understand what entanglement is, but not to have a way to prove it. Yes, it sounds confusing, but believe me, the devil is in the detail and there just isn't enough space for us to formulate a comprehensive answer to how entanglement works. But enough of that – let's get to work!
Quantum entanglement, or just entanglement, is simply defined as a quantum mechanical phenomenon that occurs when two or more particles have correlated states. What this, in essence, means is that if you have two particles or, for our purposes, qubits, that are entangled, this means that when we measure one qubit, we can determine the result of the other qubit based on the measurement of the first qubit.
As you may recall from our previous example, if we put a qubit in a superposition and we measure that qubit, we have a 50/50 split as to whether that qubit would collapse to either of two states, or
.
Now, if that same qubit were entangled with another qubit and we were to measure one of the qubits, that qubit will be either or
. However, if we were to measure the second qubit, either at the exact same time or sometime later, it too will have the same value as the first qubit we measured!
You're probably thinking, how can this be? If we take two qubits and place them in superposition and we measure them separately, we will correctly see that each qubit will collapse to a value of 1 or 0, where each time we measure the qubits individually, it may not collapse to the same value at the same time. This means that if we run the experiment one shot at a time, we would see that, sometimes, the first qubit will measure 0, while the second qubit could measure 0 or 1.
Both are separate and do not know the value of each other either before, during, or after measurement. However, if we were to entangle the two qubits and repeat the same experiment, we would see that the qubits will measure the exact same values each and every time!
Impossible, you say? Well, it's a good thing for us that we now have a quantum computer that we can run and try this out!
In the following code, we will see that when qubits are not entangled, their results are such that we cannot infer what the result of one qubit would be based on the result of the other qubit. Since we are measuring two qubits, our results will be listed as 2-bit values:
- First, we'll create a new circuit with two qubits, place them in superposition, and measure them:
#Create a circuit with 2 qubits and 2 classic bits
qc = QuantumCircuit(2,2)
#Add an H gate to each
qc.h(0)
qc.h(1)
#Measure the qubits to the classical bit
qc.measure([0,1],[0,1])
#Draw the circuit
qc.draw()
In the preceding code, we created a quantum circuit with two qubits, added an H gate to each of the qubits so that we can place each qubit into a superposition state, and finally added a measurement from each qubit to its respective bit.
The result from the previous code should display the following circuit, where we can see that each qubit has an H gate that's measured to its respective classical bit register; that is, qubit 0 to bit 0 and qubit 1 to bit 1:
Figure 4.9 - Two qubits in superposition and measured to their respective classic bits
- Then, we execute the circuit and display the results:
#Execute the circuit again and print the results
backend = Aer.get_backend('qasm_simulator')
result = execute(qc, backend, shots=1000).result()
counts = result.get_counts(qc)
plot_histogram(counts)
In the previous code, we created the backend to run on the simulator with 1000 shots and plot the results in a histogram to review them.
Important Note
Note from the following results that the outcomes are very random from each qubit, which is what we expected. One thing I would also like to mention regarding notation is the ordering of the qubits. When written, the order of the qubits are a little different than the bit order. In quantum notation, the first qubit is also listed on the left-hand side, while subsequent qubits are added toward the right-hand side. In binary notation, however, the first bit is on the right-hand side, while subsequent bits are added toward the left-hand side.
For example, if we want to represent the 3-qubit value of the number 5, we would do so using
, which is the same as the bit representation of the same number. However, the qubit order here is different as the first qubit is listed in the left position (q[0]), the second qubit (q[1]) is listed in the middle position, and the last qubit (q[2]) is listed in the right position.
On the other hand, in bit notation, the first bit (b[0]) is in the right position and moves up in order to the left. When measuring, we link the results from the qubit to the bit (as shown in the preceding screenshot), which correctly maps the results of each qubit to its respective binary position so that our results are in the expected bit order.
The plotted histogram is shown in the following screenshot:
Figure 4.10 - Random results of all combinations from both qubits
In the previous screenshot, each qubit has collapsed to a state of either 0 or 1, so since there are two qubits, we should expect to see all four random results, which are 00, 01, 10, and 11. Your probability results might differ a bit, but overall, they should all be close to 25% probability.
- This is expected, so let's entangle the two qubits and see what happens then. For this, we will entangle the two qubits and rerun the experiment.
Let's entangle the two qubits by adding a multi-qubit gate called a Control-NOT (CNOT) gate. Let me explain what this gate is before we include it in our circuit.
The CNOT gate is a multi-qubit gate that operates on one qubit based on the value of another. What this means is that the qubit gate has two connecting points – one called Control and another called Target. The T is generally some operator, such as a NOT (X) gate, which would flip the qubit from 0 to 1 or vice versa.
However, the Target operator can also be almost any operation, such as an H gate, a Y gate (which flips 180° around the Y axis), and so on. It could even be another Control, but we will get into those fancy gates in Chapter 6, Understanding Quantum Gates.
The CNOT gate acts in such a manner that when the qubit tied to the Control is set to 0, the value of the Target qubit does not change, meaning the Target operator will not be enabled. However, if the value of the Control qubit is 1, this will trigger the Target operator. This would, therefore, in the case of a CNOT gate, enable a NOT operation on the target qubit, causing it to flip 180° around the X axis from its current position.
The following logic table represents the Control and Target value updates based on the value of the Control for a CNOT gate, as well as the states before and after the CNOT gate:
Table 4.1 - Two qubit CNOT logic table
Now that we can see how the CNOT gate works on two qubits, we will update our circuit so that we can entangle the qubits together. In the following code, we will create a circuit with 2 qubits where we will apply a Hadamard gate to the first qubit and then entangle the first qubit with the second qubit using a CNOT gate:
#Create a circuit with 2 qubits and 2 classic bits
qc = QuantumCircuit(2,2)
#Add an H gate to just the first qubit
qc.h(0)
#Add the CNOT gate to entangle the two qubits, where the #first qubit is the Control, and the second qubit is the #Target.
qc.cx(0,1)
#Measure the qubits to the classical bit
qc.measure([0,1],[0,1])
#Draw the circuit
qc.draw()
The resulting diagram of the circuit should look as follows:
Figure 4.11 - Entanglement of two qubits
The previous screenshot shows you that, this time, we are only placing a Hadamard gate on the first qubit and leaving the second qubit to be operated on only by the CNOT gate. Since it is set as the target, it will be dependent on the Control qubit.
- Now, we will run the experiment and plot the results. This is similar to the previous experiments we completed, where we will execute the circuit, extract the result counts, and plot them on a histogram to visualize the results:
#Execute the circuit again and print the results
result = execute(qc, backend, shots=1000).result()
counts = result.get_counts(qc)
plot_histogram(counts)
The results shown in the following screenshot show two quantum computing principles – the superposition of the qubits, 0 and 1, and the entanglement – where both qubit's (Control and Target) results are strongly correlated as either 00 or 11:

Figure 4.12 - Results of two entangled qubits
Now that you are familiar with superposition and entanglement, let's move onto the last quantum computing principle, which is interference.
Learning about the effects of interference between qubits
One of the benefits of quantum computing is its ability to interleave these principles in such a way that usually, while explaining one, you can very easily describe the other. We did this earlier in this chapter with respect to interference. Let's review and see where we have come across this phenomenon and its usage so far.
First, recall that, at the beginning of this chapter, we described the double-slit experiment. There, we discussed how an electron can act as both a wave and a particle. When acting like a wave, we saw that the experiment illustrated how the electrons traveled and landed at certain spots of the observation screen. The pattern that it displayed was generally one that we recognize from classic physics as wave interference.
The pattern had the probabilistic results along the backboard, as shown in the observing screen in Figure 4.2, where the center of the screen has the highest number of electrons and the blank areas along both sides had least to none. This is due to the constructive and destructive interference of the waves.
There are two types of interference, namely, constructive and destructive. Constructive interference occurs when the peaks of two waves are summed up where the resulting amplitude is equal to the total positive sum of the two individual waves.
Destructive interference occurs similar to constructive interference except that the amplitudes of the waves are opposite in that when summing them together, the two waves cancel each other out.
The following diagram illustrates the constructive and destructive wave interference of two waves when they are added together:

Figure 4.13 – Constructive (left) and destructive (right) wave interferences (image source: https://commons.wikimedia.org/wiki/File:Interference_of_two_waves.svg)
The preceding diagram illustrates how two waves interfere with each other constructively and destructively. The two waves toward the bottom of the diagram represent the individual amplitudes of each wave, while the top line represents the added amplitude values, which represent the result of the interference between the two waves.
Now that you understand the difference between constructive and destructive interference, how can we apply this to what we've learned so far? Well, if you recall, earlier, when we placed a qubit in superposition, we had two distinct results.
One was from the basis state , while the other was from the basis state
. Do you remember when we started at either of these two qubit basis states, where on the X-axis of the qubit the Hadamard landed? From
, it would land on the positive side of the X axis, but, if we placed the qubit into superposition starting from the
state, it would land on the negative X axis.
Having the ability to place the qubit state vector on either the positive or negative X-axis provides us with a way to place the qubit in either a positive or negative state. Very similar to the waves in the preceding diagram, which have positive (peaks) and negative (toughs) amplitudes, qubits can also represent similar states. Let's simplify this by re-introducing the two Dirac notation values, , and
, where the
state represents the state vector on the positive X axis and the
state represents the state vector on the negative X-axis.
These new vector definitions, which represent the vector state of a qubit in superposition, will be used by some of the algorithms as a technique to identify certain values and react to them using interference – techniques such as amplitude estimation or search algorithms such as Grover's algorithm.
We can't finish this chapter without at least putting all these things together to see how these all interact in a simple example, which we will see in the next section.