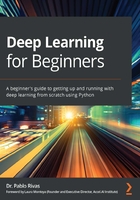
Introduction and setup of Keras
If you search on the internet for sample TensorFlow code, you will find that it may not be super easy to understand or follow. You can find tutorials for beginners but, in reality, things can get complicated very easily and editing someone else's code can be very difficult. Keras comes as an API solution to develop deep learning Tensorflow model prototypes with relative ease. In fact, Keras supports running not only on top of TensorFlow, but also over CNTK and Theano.
We can think of Keras as an abstraction to actual TensorFlow models and methods. This symbiotic relationship has become so popular that TensorFlow now unofficially encourages its use for those who are beginning to use TensorFlow. Keras is very user friendly, it is easy to follow in Python, and it is easy to learn in a general sense.
Setup
To set up Keras on your Colab, do the following:
- Run the following command:
!pip install keras
- The system will proceed to install the necessary libraries and dependencies. Once finished, type and run the following code snippet:
import keras
print(keras.__version__)
This outputs a confirmation message of it using TensorFlow as the backend as well as the latest version of Keras, which at the time of writing this book is 2.2.4. Thus, the output looks like this:
Using TensorFlow backend.
2.2.4
Principles behind Keras
There are two major ways in which Keras provides functionality to its users: a sequential model and the Functional API.
These can be summarized as follows:
- Sequential model: This refers to a way of using Keras that allows you to linearly (or sequentially) stack layer instances. A layer instance, in this case, has the same meaning as in our previous discussions in Chapter 1, Introduction to Machine Learning. That is, a layer has some type of input, some type of behavior or main model operation, and some type of output.
- Functional API: This is the best way to go deeper in defining more complex models, such as merge models, models with multiple outputs, models with multiple shared layers, and many other possibilities. Don't worry, these are advanced topics that will become clear in further chapters. The Functional API paradigm gives the coder more freedom to do different innovative things.
We can think of the sequential model as an easy way of starting with Keras, and the Functional API as the way to go for more complex problems.
Remember the shallow neural network from Chapter 1, Introduction to Machine Learning? Well, this is how you would do that model using the sequential model paradigm in Keras:
from keras.models import Sequential
from keras.layers import Dense, Activation
model = Sequential([
Dense(10, input_shape=(10,)),
Activation('relu'),
Dense(8),
Activation('relu'),
Dense(4),
Activation('softmax'),
])
The first two lines of code import the Sequential model and the Dense and Activation layers, respectively. A Dense layer is a fully connected neural network, whereas an Activation layer is a very specific way of invoking a rich set of activation functions, such as ReLU and SoftMax, as in the previous example (these will be explained in detail later).
Alternatively, you could do the same model, but using the add() method:
from keras.models import Sequential
from keras.layers import Dense, Activation
model = Sequential()
model.add(Dense(10, input_dim=10))
model.add(Activation('relu'))
model.add(Dense(8))
model.add(Activation('relu'))
model.add(Dense(4))
model.add(Activation('softmax'))
This second way of writing the code for the neural model looks more linear, while the first one looks more like a Pythonic way to do so with a list of items. It is really the same thing and you will probably develop a preference for one way or the other. However, remember, both of the previous examples use the Keras sequential model.
Now, just for comparison purposes, this is how you would code the exact same neural network architecture, but using the Keras Functional API paradigm:
from keras.layers import Input, Dense
from keras.models import Model
inputs = Input(shape=(10,))
x = Dense(10, activation='relu')(inputs)
x = Dense(8, activation='relu')(x)
y = Dense(4, activation='softmax')(x)
model = Model(inputs=inputs, outputs=y)
If you are an experienced programmer, you will notice that the Functional API style allows more flexibility. It allows you to define input tensors to use them as input to different pieces of the model, if needed. However, using the Functional API does assume that you are familiar with the sequential model. Therefore, in this book, we will start with the sequential model and move forward with the Functional API paradigm as we make progress toward more complex neural models.
Just like Keras, there are other Python libraries and frameworks that allow us to do machine learning with relatively low difficulty. At the time of writing this book, the most popular is Keras and the second most popular is PyTorch.