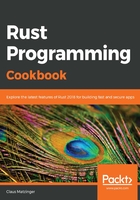
上QQ阅读APP看书,第一时间看更新
How to do it...
Compile your code comments to a shiny HTML in just a few steps:
- Rust's docstrings (strings that explicitly are documentation to be rendered) are denoted by /// (instead of the regular //). Within these sections, markdown—a shorthand language for HTML—can be used to create full documentation. Let's add the following before the List<T> declaration:
///
/// A singly-linked list, with nodes allocated on the heap using
///`Rc`s and `RefCell`s. Here's an image illustrating a linked list:
///
///
/// 
///
/// *Found on https://en.wikipedia.org/wiki/Linked_list*
///
/// # Usage
///
/// ```
/// let list = List::new_empty();
/// ```
///
#[derive(Clone)]
pub struct List<T> where T: Sized + Clone {
[...]
- This makes the code a lot more verbose, but is this worth it? Let's see with cargo doc, a subcommand that runs rustdoc on the code and outputs HTML in the target/doc directory of the project. When opened in a browser, the target/doc/testing/index.html page shows the following (and more):

Replace testing with the name of your project!
- Great, let's add more documentation in the code. There are even special sections that are recognized by the compiler (by convention):
///
/// Appends a node to the list at the end.
///
///
/// # Panics
///
/// This never panics (probably).
///
/// # Safety
///
/// No unsafe code was used.
///
/// # Example
///
/// ```
/// use testing::List;
///
/// let mut list = List::new_empty();
/// list.append(10);
/// ```
///
pub fn append(&mut self, value: T) {
[...]
- The /// comments add documentation for expressions that follow it. This is going to be a problem for modules: should we put the documentation outside of the current module? No. Not only will this make the maintainers confused, but it also has a limit. Let's use //! to document the module from within:
//!
//! A simple singly-linked list for the Rust-Cookbook by Packt
//! Publishing.
//!
//! Recipes covered in this module:
//! - Documenting your code
//! - Testing your documentation
//! - Writing tests and benchmarks
//!
- A quick cargo doc run reveals whether it worked:

- While there is some benefit in having similar-looking documentation in any Rust project, corporate marketing often likes to have things such as logos or a custom favicon to stand out. rustdoc supports that with attributes on the module level—they can be added right below the module documentation (note: this is the logo of my Rust blog, https://blog.x5ff.xyz):
#![doc(html_logo_url = "https://blog.x5ff.xyz/img/main/logo.png")]
- To see whether it worked, let's run cargo doc again:

Now, let's go behind the scenes to understand the code better.