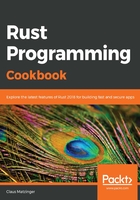
上QQ阅读APP看书,第一时间看更新
How to do it...
The steps for this recipe are as follows:
- Use cargo to create a new project, cargo new sequences --lib, or clone it from this book's GitHub repository (https://github.com/PacktPublishing/Rust-Programming-Cookbook). Use Visual Studio Code and Terminal to open the project's directory.
- With the test module ready, let's start with arrays. Arrays in Rust have a familiar syntax but they follow a stricter definition. We can try out various abilities of the Rust array in a test:
#[test]
fn exploring_arrays() {
let mut arr: [usize; 3] = [0; 3];
assert_eq!(arr, [0, 0, 0]);
let arr2: [usize; 5] = [1,2,3,4,5];
assert_eq!(arr2, [1,2,3,4,5]);
arr[0] = 1;
assert_eq!(arr, [1, 0, 0]);
assert_eq!(arr[0], 1);
assert_eq!(mem::size_of_val(&arr), mem::size_of::<usize>()
* 3);
}
- Users of more recent programming languages and data science/math environments will also be familiar with the tuple, a fixed-size variable type collection. Add a test for working with tuples:
struct Point(f32, f32);
#[test]
fn exploring_tuples() {
let mut my_tuple: (i32, usize, f32) = (10, 0, -3.42);
assert_eq!(my_tuple.0, 10);
assert_eq!(my_tuple.1, 0);
assert_eq!(my_tuple.2, -3.42);
my_tuple.0 = 100;
assert_eq!(my_tuple.0, 100);
let (_val1, _val2, _val3) = my_tuple;
let point = Point(1.2, 2.1);
assert_eq!(point.0, 1.2);
assert_eq!(point.1, 2.1);
}
- As the last collection, the vector is the basis for all of the other quick and expandable data types. Create the following test with several assertions that show how to use the vec! macro and the vector's memory usage:
use std::mem;
#[test]
fn exploring_vec() {
assert_eq!(vec![0; 3], [0, 0, 0]);
let mut v: Vec<i32> = vec![];
assert_eq!(mem::size_of::<Vec<i32>>(),
mem::size_of::<usize>
() * 3);
assert_eq!(mem::size_of_val(&*v), 0);
v.push(10);
assert_eq!(mem::size_of::<Vec<i32>>(),
mem::size_of::<i32>() * 6);
The remainder of the test shows how to modify and read the vector:
assert_eq!(v[0], 10);
v.insert(0, 11);
v.push(12);
assert_eq!(v, [11, 10, 12]);
assert!(!v.is_empty());
assert_eq!(v.swap_remove(0), 11);
assert_eq!(v, [12, 10]);
assert_eq!(v.pop(), Some(10));
assert_eq!(v, [12]);
assert_eq!(v.remove(0), 12);
v.shrink_to_fit();
assert_eq!(mem::size_of_val(&*v), 0);
}
- Run cargo test to see the working tests run:
$ cargo test
Compiling sequences v0.1.0 (Rust-Cookbook/Chapter01/sequences)
Finished dev [unoptimized + debuginfo] target(s) in 1.28s
Running target/debug/deps/sequences-f931e7184f2b4f3d
running 3 tests
test tests::exploring_arrays ... ok
test tests::exploring_tuples ... ok
test tests::exploring_vec ... ok
test result: ok. 3 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Doc-tests sequences
running 0 tests
test result: ok. 0 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Now, let's go behind the scenes to understand the code better.