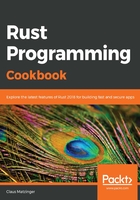
How it works...
Options are, to our initial surprise, an enum. While this almost guarantees great match compatibility, enums behave a lot like structs in the remaining aspects. In Step 2, we see that it's not just a regular enum, but also a typed enum—which forces us to add a type declaration to None as well. Step 2 also shows ways how to get values out of the Option type, with and without panicking. unwrap() is a popular choice, but it comes with some variations that don't halt the thread if None is encountered.
unwrap() is always a dangerous thing, and should only be used in non-production code. It panics, which can bring the entire program to a sudden, unexpected halt, and doesn't even leave you with a proper error message. If stopping the program is the desired outcome, expect() is a better choice since it lets you add a simple message. This is why we added the #[should_panic] attribute to the unit test, so that we can prove to you that it actually panics (or otherwise the test fails).
Step 3 shows some non-intrusive ways to unwrap the value of Option. Especially since unwrap() returns the owned value while destroying Option itself, other ways can be more useful if Option remains part of a data structure and only temporarily holds a value. take() was designed for these situations that replace the value with None, similar to replace(), which does the same for the replacement value. Furthermore, there is map(), which lets you work directly with the value (if present) and ignore the usual if-then or match constructs, which add a lot of code verbosity (refer to step 5).
Step 4 features an interesting tidbit in the middle: Options can be used like Booleans to perform logic operations, similar to Python, where AND/OR operations return a particular operand (https://docs.python.org/3/reference/expressions.html#boolean-operations) in either case. Last but not least, Options can also be treated like collections using an iterator.
Rust's options are very versatile and, by looking at the documents (https://doc.rust-lang.org/std/option/index.html), you can find out many different ways to transform values on the fly without tedious guard clauses with if, let, and match.
Now that we have successfully learned that there is no null in Rust, let's move on to the next recipe.