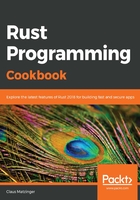
How it works...
Iterators are a great way of providing advanced capabilities to custom data structures. With their simple, unified interface, collection types can be switched out easily as well and programmers don't have to get used to new APIs for every data structure.
By implementing the Iterator trait in Steps 1 and 2, it becomes easy to provide exactly the desired access level to a collection's elements. In the case of this recipe (and similar to Vec<T>), it will consume the list entirely and remove the items one by one, starting at the front.
In Step 3, we implement IntoIterator, a trait that makes this construct available to the for loop and other users who call into_iter(). Not every collection implements this trait to provide multiple different iterators; for example, the second iterator of Vec<T> is reference-based, and only accessible via an iter() function on the type. By the way, a reference is a data type, just like the actual instance, so it's all about the type definition in this case. These definitions are made inside the trait implementation with the type Item declaration (so-called associated types: https://doc.rust-lang.org/rust-by-example/generics/assoc_items/types.html). These types are called associated types, and can be referenced using Self::Item—just like generics, but without the added syntax verbosity.
With these interfaces, you can get access to a large library of functions that only assume a working iterator to be present! Check out Steps 4 and 5 to see the implementation and outcome of using the iterator on a newly created list type.
We've successfully learned how to implement custom iterators. Now, let's move on to the next recipe.