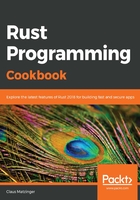
How it works...
While unsafe is one way to enable easier solutions to sometimes tricky situations, this book (https://rust-unofficial.github.io/too-many-lists/index.html) describes the limitations of safe programming perfectly with something as simple as a linked list.
After setting up the project in Step 1, we are adding the first function in Step 2. Its purpose is similar to chunks() (https://doc.rust-lang.org/std/primitive.slice.html#method.chunks_mut), but instead of an iterator, we are returning the entire collection right away, which is OK as an example, but should be thought about when implementing it for production use. Our function splits a provided (mutable) slice into a parts number of chunks of equal size and returns mutable references to them. Since the input is also a mutable reference to the entire part of the memory, we will have parts + 1 number of mutable references to the same memory area; clearly, a violation of safe Rust! On top of that, this function allows going beyond the allocated memory with the ptr.offset() call (which does pointer arithmetic).
In the test created in Step 3, we show that it compiles and executes without any major problems. Step 4 provides another example for unsafe code: changing the data type without casting. The transmute (https://doc.rust-lang.org/std/mem/fn.transmute.html) function can easily change the data type of a variable with all the consequences that come with that. Had we changed the type to something else, such as u64, we would end up with a totally different result and read memory that does not belong to the program. In Step 5, we run the whole test suite.
unsafe Rust can be interesting to get that last bit of performance out of a data structure, do some magic bin-packing, or to implement Send and Sync (https://doc.rust-lang.org/std/mem/fn.transmute.html). Whatever you intend to do with unsafe, check out the nomicon (https://doc.rust-lang.org/nightly/nomicon/) to delve deeper into the depths.
Equipped with that knowledge, let's move on to the next recipe.