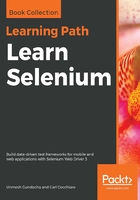
Stream.min() and Stream.max()
The Streams API provides min() and max() methods—stream processing for finding the minimum or maximum value in the stream respectively.
Let's take an example in the context of the sample application we're testing. We will create a simple Java class called Product that stores the name and price of products returned by the search. We want to find the product that has the minimum price and the one that has the maximum price. Our product class will have two members, as shown in the following code:
class Product {
String name;
Double price;
public Product(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public Double getPrice() {
return price;
}
}
Let's create a list of products returned by the search result, as shown here:
List<Product> searchResult = new ArrayList<>();
searchResult.add(new Product("MADISON OVEREAR HEADPHONES", 125.00));
searchResult.add(new Product("MADISON EARBUDS", 35.00));
searchResult.add(new Product("MP3 PLAYER WITH AUDIO", 185.00));
We can call the .min() function by passing the comparison attribute, in this case, price, using the .getPrice() method. The .min() function will use the price attribute and return the element that has the lowest price, as shown in this code:
Product product = searchResult.stream()
.min(Comparator.comparing(item -> item.getPrice()))
.get();
System.out.println("The product with lowest price is " + product.getName());
The get() method will return the object returned by the min() function. We will store this in an instance of Product. The min() function finds MADISON EARBUDS as the lowest-priced product, as shown in the following console output:
The product with lowest price is MADISON EARBUDS
As opposed to the min() function, the max() function will return the product with the highest price, as shown in the following code:
product = searchResult.stream()
.max(Comparator.comparing(item -> item.getPrice()))
.get();
System.out.println("The product with highest price is " + product.getName());
The max() function finds MP3 PLAYER WITH AUDIO as the highest-priced product:
The product with highest price is MP3 PLAYER WITH AUDIO
The min() and max() functions return an optional instance, which has a get() method to obtain the object. The get() method will return null if the stream has no elements.
Both the functions take a comparator as a parameter. The Comparator.comparing() method creates a comparator based on the lambda expression passed to it.