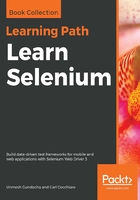
上QQ阅读APP看书,第一时间看更新
Filtering and performing actions on WebElements
Let's further modify the search test and find a product matching with a given name. We will then click on the product to open the product details page, as shown in this code:
@Test
public void searchAndViewProduct() {
// find search box and enter search string
WebElement searchBox = driver.findElement(By.name("q"));
searchBox.sendKeys("Phones");
WebElement searchButton =
driver.findElement(By.className("search-button"));
searchButton.click();
assertThat(driver.getTitle())
.isEqualTo("Search results for: 'Phones'");
List<WebElement> searchItems = driver
.findElements(By.cssSelector("h2.product-name a"));
WebElement product = searchItems.stream()
.filter(item -> item.getText().equalsIgnoreCase("MADISON EARBUDS"))
.findFirst()
.get();
product.click();
assertThat(driver.getTitle())
.isEqualTo("Madison Earbuds");
}
In the preceding code, we used the filter() function to find a specific product from the list of WebElements. We retrieved the first matching product, using the findFirst() function. This will return a WebElement representing the link element. We then clicked on the element to open the product details page in the browser.
Thus, we can use Streams API in a number of ways to create functional, readable code with just a few lines.