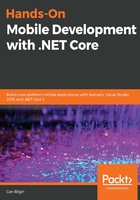
Xamarin.Forms
In the previous examples, you can easily see that Xamarin.Forms greatly simplifies the process of creating UI mobile applications on two complete different platforms using the same declarative view tree, even though the native approaches on these platforms are, in fact, almost completely different.
From a UI renderer perspective, Xamarin.Forms provides the native rendering with two different ways of using the same toolset at compile-time (compiled XAMLs) and at runtime (runtime rendering). In both scenarios, page-layout-view hierarchies that are declared in XAML layouts are rendered using renderers. Renderers can be described as the implementations of the view abstractions on target platforms. For instance, the renderer for the label element on iOS is responsible for translating label control (as well as its layout attributes) into a UILabel control.
Nevertheless, Xamarin.Forms can't just be categorized as a UI framework, since it provides various modules out of the box which are essential to most mobile application projects, such as dependency services and messenger services. Being among the main patterns for creating SOLID applications, these components provide the tools to create abstractions on platform-specific implementations, thus unifying the cross-platform architecture in order to create application logic that spans across multiple platforms.
Additionally, the Data Binding concept, which is the heart and soul of any Model-View-ViewModel (MVVM) implementation, can be directly introduced in the XAML level, saving the developers from having to create their own data synchronization logic.
For instance, if we were to expand the implementation from the previous example by creating a ViewModel for our main view, we could make use of our shared project, which was created as part of the multi-project template.
The first step here would be to create the MainPageViewModel class under the shared project that contains the view that was created previously. Inside this class, we will create a single (get-only) property, which we will use in our view:
namespace FirstXamarinFormsApplication
{
public class MainPageViewModel
{
public MainPageViewModel()
{
}
public string Platform
{
get
{
#if __IOS__
return "iOS";
#elif __ANDROID__
return "Android";
#endif
}
}
}
}
Now, let's assign this view model as our binding context on the main view:
namespace FirstXamarinFormsApplication
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
BindingContext = new MainPageViewModel();
}
}
}
Then, we will update our XAML to use the bound view model data:

In this example, our binding of the label view looks similar to the following:
<Label Text="{Binding Platform, StringFormat='Welcome to {0}!'}"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand" />
Using data bindings can substantially decrease the complexity of your applications, creating highly robust and maintainable mobile applications.