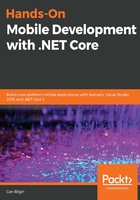
上QQ阅读APP看书,第一时间看更新
Attached properties
Another way to implement behaviors in order to modify default control behavior is to use attached properties to declare a bindable extension to existing controls. This approach is generally used for small behavioral adjustments, such as enabling/disabling other behaviors, as well as adding/removing effects. Let's get started:
- In order to implement such a behavior, we need to create a bindable property that will be used on other controls:
public static class Validations
{
public static readonly BindableProperty ValidateRequiredProperty =
BindableProperty.CreateAttached(
"ValidateRequired",
typeof(bool),
typeof(RequiredValidation),
false,
propertyChanged: OnValidateRequiredChanged);
public static bool GetValidateRequired(BindableObject view)
{
return (bool)view.GetValue(ValidateRequiredProperty);
}
public static void SetValidateRequired(BindableObject view,
bool value)
{
view.SetValue(ValidateRequiredProperty, value);
}
private static void OnValidateRequiredChanged(
BindableObject bindable, object oldValue, object
newValue)
{
// TODO:
}
}
- In the case of attached behaviors, the static class can be directly accessed so that it sets the attached property on the current control (instead of creating and adding a behavior):
<Entry x:Name="usernameEntry" Placeholder="username"
Text="{Binding UserName, Mode=OneWayToSource}"
behaviors:Validations.ValidateRequired="true" >
- By implementing the ValidateRequired property changed handler, we can have the attached property insert and remove the required validation to various Entry views:
private static void OnValidateRequiredChanged(
BindableObject bindable,
object oldValue,
object newValue)
{
if(bindable is Entry entry)
{
if ((bool)newValue)
{
entry.Behaviors.Add(new ValidationBehavior()
{
ValidationRule = new RequiredValidationRule()
});
}
else
{
var behaviorToRemove = entry.Behaviors
.OfType<ValidationBehavior>()
.FirstOrDefault(
item => item.ValidationRule is
RequiredValidationRule);
if (behaviorToRemove != null)
{
entry.Behaviors.Remove(behaviorToRemove);
}
}
}
}