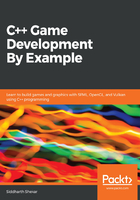
Arrays
So far, we have only looked at single variables, but what if we want a bunch of variables grouped together? Like the ages of all the students in a class, for example. You can keep creating separate variables: a, b, c, d, and so on, and to access each you would have to call each of them, which is cumbersome, as you won't know what kind of data they hold.
To organize data better, we can use arrays. Arrays use continuous memory space to store values in a series and you can access each element with an index number.
The syntax for arrays is as follows:
type name [size] = { value0, value1, ....., valuesize-1};
So, we can store the age of five students as follows:
int age[5] = {12, 6, 18 , 7, 9 };
When creating an array with a set number of values, you don't have to specify a size but it is a good idea to do so. To access each value, we use the index from 0-4 as the first element with a value of 12 at the 0th index and the last element, 9, in the fourth index.
Let's see how to use this in code:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int age[5] = { 12, 6, 18 , 7, 9 }; std::cout << "Element at the 0th index " << age[0]<< std::endl; std::cout << "Element at the 4th index " << age[4] << std::endl; _getch(); return 0; }
The output is as follows:

To access each element in the array you can use the for loop:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int age[5] = { 12, 6, 18 , 7, 9 }; for (int i = 0; i < 5; i++) { std::cout << "Element at the "<< i << "th index is: " <<
age[i] << std::endl; } _getch(); return 0; }
The output of this is as follows:

Instead of calling age[0], and so on, we use the i index from the for loop itself and pass it into the age array to print out the index and the value stored at that index.
The age array is a single-dimension array. In graphics programming, we have seen that we use a two-dimensional array, which is mostly a 4x4 matrix. Let's look at an example of a two-dimensional 4x4 array. A two-dimensional array is defined as follows:
int matrix[4][4] = {
{2, 8, 10, -5},
{15, 21, 22, 32},
{3, 0, 19, 5},
{5, 7, -23, 18}
};
To access each element, you will use a nested for loop.
Let's look at this in the following code:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { int matrix[4][4] = { {2, 8, 10, -5}, {15, 21, 22, 32}, {3, 0, 19, 5}, {5, 7, -23, 18} }; for (int x = 0; x < 4; x++) { for (int y = 0; y < 4; y++) { std::cout<< matrix[x][y] <<" "; } std::cout<<""<<std::endl; } _getch(); return 0; }
The output is as follows:

As a test, create two matrices and attempt to carry out matrix multiplication.
You can even pass arrays as parameters to functions, shown as follows.
Here, the matrixPrinter function doesn't return anything but prints out the values stored in each element of the 4x4 matrix:
#include <iostream> #include <conio.h> void matrixPrinter(int a[4][4]) { for (int x = 0; x < 4; x++) { for (int y = 0; y < 4; y++) { std::cout << a[x][y] << " "; } std::cout << "" << std::endl; } } // Program prints out values to screen int main() { int matrix[4][4] = { {2, 8, 10, -5}, {15, 21, 22, 32}, {3, 0, 19, 5}, {5, 7, -23, 18} }; matrixPrinter(matrix); _getch(); return 0; }
We can even use an array of char to create a string of words. Unlike int and float arrays, the characters in an array don't have to be in curly brackets and they don't need to be separated by a comma.
To create a character array, you will define it as follows:
char name[] = "Hello, World !";
You can print out the values just by calling out the name of the array, as follows:
#include <iostream> #include <conio.h> // Program prints out values to screen int main() { char name[] = "Hello, World !"; std::cout << name << std::endl; _getch(); return 0; }
The output of this is as follows:
