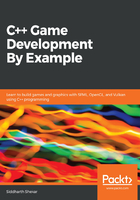
Structs
Structures or structs are used to group data together. A struct can have different data elements in it, called members, integers, floats, chars, and so on. You can create many objects of a similar struct and store values in the struct for data management.
The syntax of a struct is shown as follows:
struct name{ type1 name1; type2 name2; . . } ;
An object of a struct can be created as follows:
struct_name object_name;
An object is an instance of a struct to which we can assign properties to the data types we created when creating the struct. An example of this is as follows.
In a situation in which you want to maintain a database of students' ages and the height of a section, your struct definition will look like this:
struct student { int age; float height; };
Now you can create an array of objects and store the values for each student:
int main() { student section[3]; section[0].age = 17; section[0].height = 39.45f; section[1].age = 12; section[1].height = 29.45f; section[2].age = 8; section[2].height = 13.45f; for (int i = 0; i < 3; i++) { std::cout << "student " << i << " age: " << section[i].age << " height: " << section[i].height << std::endl; } _getch(); return 0; }
Here is the output of this:
