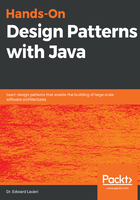
Overloading constructors and methods
When constructors and methods are declared, we include the expected parameters. For example, our Bicycle() constructor does not take any parameters. We can overload that constructor by creating one or more versions of it, each with a different set of parameters. Let's look at our Bicycle class with four constructors:
// constructor - default
Bicycle() {
}
// constructor - String parameter
Bicycle(String aColor) {
this.color = aColor;
}
// constructor - int parameter
Bicycle(int nbrOfGears) {
this.gears = nbrOfGears;
}
// constructor - int, double, double, String parameters
Bicycle(int nbrOfGears, double theCost, double theWeight, String aColor) {
this.gears = nbrOfGears;
this.cost = theCost;
this.weight = theWeight;
this.color = aColor;
}
The default constructor is used when an instance of the object is created without any parameters. We would use the following code link:
Bicycle myBike1 = new Bicycle();
We can use one of the overloaded methods when creating a Bicycle object, simply by passing the proper arguments, based on what the overloaded method expects as parameters. The following Driver class shows the creation of four Bicycle objects, one using a different constructor:
public class Driver {
public static void main(String[] args) {
Bicycle myBike1 = new Bicycle();
Bicycle myBike2 = new Bicycle("Brown");
Bicycle myBike3 = new Bicycle(22);
Bicycle myBike4 = new Bicycle(22, 319.99, 13.5, "White");
myBike1.outputData();
myBike2.outputData();
myBike3.outputData();
myBike4.outputData();
}
}
As you can see from the following output provided, each Bicycle object was created with a different constructor. Overloading the constructor increases the flexibility in object creation:

We can also overload methods. For example, we have used the outputData() method in our Bicycle class several times already. Let's overload that method so that we can pass additional text to be printed in the output. Here are both versions of that method:
// method to output Bicycle's information
public void outputData() {
System.out.println("\nBicycle Details:");
System.out.println("Gears : " + this.gears);
System.out.println("Cost : " + this.cost);
System.out.println("Weight : " + this.weight + " lbs");
System.out.println("Color : " + this.color);
}
// method to output Bicycle's information - overloaded
public void outputData(String bikeText) {
System.out.println("\nBicycle " + bikeText + " Details:");
System.out.println("Gears : " + this.gears);
System.out.println("Cost : " + this.cost);
System.out.println("Weight : " + this.weight + " lbs");
System.out.println("Color : " + this.color);
}
As you can see in the preceding code, our overloaded method accepts a String parameter and incorporates that in the first printed line. We can modify our Driver class as shown in the following code snippet:
myBike1.outputData("Nbr 1");
myBike2.outputData("Nbr 2");
myBike3.outputData("Nbr 3");
myBike4.outputData("Nbr 4");
The output is presented here and illustrates how easy it is to create and use overloaded methods:
