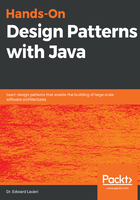
Being purposeful with inheritance
Inheritance is a powerful OOP construct. We can model objects efficiently, as was illustrated with the bicycle. We know that the following relationships exist:
- Bicycle is a two wheeled
- Bicycle is a vehicle
- Bicycle is a object
When we program inheritance, we should perform the "IS A" Checks using the following pseudo-code logic:
if ( <new child object> is a <parent object> ) then relationship = True; else relationship = False
If our "IS A" Checks fails, then inheritance should be avoided. This is an important test that, with dedicated use, can help ensure that inheritance lines between objects are valid.
Let's create the "IS A" Checks for our Bicycle class. We will do this by using Java's instanceof operator. Here is the code in three sections. The first section runs the checks for myBike6 and checks to see whether it is an instance of Bicycle, TwoWheeled, Vehicle, and Object:
// "IS A" Checks
System.out.println("\n\"IS A\" CHECKS");
// focus on myBike6
Bicycle myBike6 = new Bicycle();
if (myBike6 instanceof Bicycle)
System.out.println("myBike6 Instance of Bicycle: True");
else
System.out.println("myBike6 Instance of Bicycle: False");
if (myBike6 instanceof TwoWheeled)
System.out.println("myBike6 Instance of TwoWheeled: True");
else
System.out.println("myBike6 Instance of TwoWheeled: False");
if (myBike6 instanceof Vehicle)
System.out.println("myBike6 Instance of Vehicle: True");
else
System.out.println("myBike6 Instance of Vehicle: False");
if (myBike6 instanceof Object)
System.out.println("myBike6 Instance of Object: True");
else
System.out.println("myBike6 Instance of Object: False");
The second section runs the checks for myTwoWheeled and checks to see whether it is an instance of Bicycle, TwoWheeled, Vehicle, and Object:
// focus on TwoWheeled
TwoWheeled myTwoWheeled = new TwoWheeled();
if (myTwoWheeled instanceof Bicycle)
System.out.println("\nmyTwoWheeled Instance of Bicycle: True");
else
System.out.println("\nmyTwoWheeled Instance of Bicycle: False");
if (myTwoWheeled instanceof TwoWheeled)
System.out.println("myTwoWheeled Instance of TwoWheeled: True");
else
System.out.println("myTwoWheeled Instance of TwoWheeled: False");
if (myTwoWheeled instanceof Vehicle)
System.out.println("myTwoWheeled Instance of Vehicle: True");
else
System.out.println("myTwoWheeled Instance of Vehicle: False");
if (myTwoWheeled instanceof Object)
System.out.println("myTwoWheeled Instance of Object: True");
else
System.out.println("myTwoWheeled Instance of Object: False");
The third and final section runs the checks for myVehicle and checks to see whether it is an instance of Bicycle, TwoWheeled, Vehicle, and Object:
// focus on Vehicle
Vehicle myVehicle = new Vehicle();
if (myVehicle instanceof Bicycle)
System.out.println("\nmyVehicle Instance of Bicycle: True");
else
System.out.println("\nmyVehicle Instance of Bicycle: False");
if (myVehicle instanceof TwoWheeled)
System.out.println("myVehicle Instance of TwoWheeled: True");
else
System.out.println("myVehicle Instance of TwoWheeled: False");
if (myVehicle instanceof Vehicle)
System.out.println("myVehicle Instance of Vehicle: True");
else
System.out.println("myVehicle Instance of Vehicle: False");
if (myVehicle instanceof Object)
System.out.println("myVehicle Instance of Object: True");
else
System.out.println("myVehicle Instance of Object: False");
The output of the three sections of is a code is provided here. As you can see, the myBike6 object is an instance of Bicycle, TwoWheeled, Vehicle, and Object; the myTwoWheeled object is an instance of TwoWheeled, Vehicle, and Object; and the myVehicle object is an instance of Vehicle and Object. We can also see that the myTwoWheeled object is not an instance of Vehicle or Object; and that the myVehicle object is not an instance of Bicycle or TwoWheeled:

The preceding screenshot depicts this example.