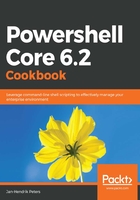
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- Static members do not require an object. To use them, however, we still need to reference the class we are using a member from. To access a method, the type name needs to inserted into square brackets and a double colon needs to be used:
# Using a static method
[Console]::Beep(440, 1000)
# Accessing a static property
[System.IO.Path]::PathSeparator
- Get-Member can help discover static members, as well with the Static parameter:
# Using Get-Member to find static properties of .NET types
# of objects
Get-Process | Get-Member -Static
[System.Diagnostics.Process]::GetCurrentProcess()
# and of types
[datetime] | Get-Member -Static
[datetime]::Parse('31.12.2018',[cultureinfo]::new('de-de'))
[datetime]::IsLeapYear(2020) # Finally, February 29th is back!
- There are many helpful .NET classes that you can use to your advantage, for example, the path class in the System.IO namespace:
# The path class is very helpful
$somePath = '/some/where/over/the.rainbow'
[IO.Path]::GetExtension($somePath)
[IO.Path]::GetFileNameWithoutExtension($somePath)
[IO.Path]::GetTempFileName() # Which is where your New-TemporaryFile is coming from
[IO.Path]::IsPathRooted($somePath)
- File and Directory classes can be used to accomplish most file- and directory-related tasks:
# Ever wanted to test your monitoring?
$file = [IO.File]::Create('.\superlarge')
$file.SetLength(1gb)
$file.Close() # Close the open handle
Get-Item $file.Name # The length on disk is now 1gb
Remove-Item $file.Name
# In order to lock a file for your script, the file class offers methods as well
$tempfile = New-TemporaryFile
$fileHandle = [IO.File]::OpenWrite($tempfile.FullName)
# Try to add content now - the file is locked
'test' | Add-Content $tempfile.FullName
# The method Write can be used now as long as you keep the handle
# Using another static method to get the bytes in a given string
$bytes = [Text.Encoding]::utf8.GetBytes('Hello :)')
$fileHandle.Write($bytes)
$fileHandle.Close() # Release the lock again
Get-Content -Path $tempfile.FullName
- The DriveInfo class can be used with the GetDrives method to retrieve all enabled drives:
# Ever needed to validate drives?
[IO.DriveInfo]::GetDrives()
if (-not $IsWindows)
{
[IO.DriveInfo]'/'
[IO.DriveInfo]'/sausage' # A valid, but non existent drive will show IsReady = $false
}
else
{
[IO.DriveInfo]'C'
[IO.DriveInfo]'Y' # A valid, but non existent drive will show IsReady = $false
}
- Other static properties can even affect the behavior of cmdlets, such as Invoke-WebRequest and Invoke-RestMethod:
$originalProtocol = [Net.ServicePointManager]::SecurityProtocol
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls12
[Net.ServicePointManager]::SecurityProtocol = $originalProtocol