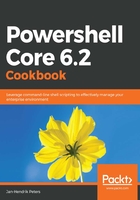
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- We will start by creating a fully-fledged .NET class to store information in. To do this, start with a new file with the ending .cs. This will be the starting point for your own class library.
- Next, add the three classes you will find in 05 - ClassesDotNet.cs to your new, empty C# file, or simply use this file:
using System.Collections.Generic;
namespace com.contoso
{
public enum DeviceType
{ }
public class Device
{ }
public class Desktop : Device
{ }
public class Server : Device
{ }
}
- By using Add-Type, you can load the library into your current session:
# Compile and import C# code on the fly
Add-Type -Path '.\05 - ClassesDotNet.cs'
- As an alternative, you can use Add-Type to compile simple libraries:
# Or save the compiled result for posterity
Add-Type -Path '.\05 - ClassesDotNet.cs' -OutputAssembly Device.dll
Add-Type -Path .\Device.dll
- By using PowerShell classes, we can be more flexible. Refer to 05 - Classes.ps1 and load the classes into memory by highlighting them and pressing F8 in an editor (or the hotkey that is bound to Run Selection):
enum DeviceType
{ }
class Device
{ }
class Desktop : Device
{ }
class Server : Device
{ }
- By using parameter validation, we can apply some additional checks to our parameter values:
[ValidatePattern('(EMEA|APAC)_\w{2}_\w{3,5}')]
[string] $Location
[ValidateSet('dev.contoso.com','qa.contoso.com','contoso.com')]
[string] $DomainName
- You can instantiate both classes by calling their constructors. Remember to use Get-Member to view the parameters that the constructor accepts:
# Instantiate classes with New-Object or the new() method
$desktop = New-Object -TypeName com.contoso.Desktop -ArgumentList 'AssetTag1234','Desktop'
$serverDotNet = New-Object -TypeName com.contoso.Server -ArgumentList 'AssetTag5678','EMEA_DE_DUE', 'contoso.com','Server'
$serverPsClass = [Server]::new('AssetTag5678','EMEA_DE_DUE', 'contoso.com','Server')
- Using the Get-Member cmdlet on both objects shows slight differences:
# Notice that our members look a bit different
# IsDomainJoined cannot be marked private for our PowerShell Class
$serverDotNet, $serverPsClass | Get-Member -Name IsDomainJoined
# While our .NET class does not show its private methods
# our PowerShell class cannot hide its hidden method any longer
$serverDotNet, $serverPsClass | Get-Member -Force -MemberType Methods