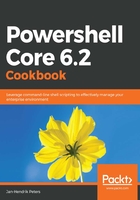
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- Custom objects often make use of the PSCustomObject data type. To create a new custom object, you can use the type-casting operator to cast a hashtable into a PSCustomObject:
# Use the typecasting operator to create one
[PSCustomObject]@{
DeviceType = 'Server'
DomainName = 'dev.contoso.com'
IsDomainJoined = $true
}
# Alternatively, New-Object might be used
New-Object -TypeName pscustomobject -Property @{
DeviceType = 'Server'
DomainName = 'dev.contoso.com'
IsDomainJoined = $true
}
- Custom objects only have the properties you have defined. Have a look at the output that Get-Member will now show:
# Using Get-Member with the output again shows more
# Notice the methods that exist, even though they have not been implemented by you?
# PSCustomObject, like many classes, inherits from the class Object
[PSCustomObject]@{
DeviceType = 'Server'
DomainName = 'dev.contoso.com'
IsDomainJoined = $true
} | Get-Member
- When Get-Member is used in the pipeline, custom objects have no immediate disadvantage:
# Provided you supply the correct property names, you can use these custom
# objects in the pipeline as well.
Get-Help Get-Item -Parameter Path
[pscustomobject]@{ Path = '/'} | Get-Item
- Using custom objects for CSV export can be a good way to share data with people who are unfamiliar with PowerShell:
# Especially when being exported, the custom object really shines
$someLogMessages = 1..10 | ForEach-Object {
[pscustomobject]@{
ComputerName = 'HostA', 'HostB', 'HostC' | Get-Random
EntryType = 'Error', 'Warning', 'Info' | Get-Random
Message = "$_ things happened today"
}
}
$someLogMessages | Export-Csv -Path .\NiceExport.csv
psedit .\NiceExport.csv
- Have a look at the data type of the objects that have been imported from any CSV file:
# When importing any csv, examine the data type
Get-Date | Export-Csv .\date.csv
Import-Csv .\date.csv | Get-Member
Remove-Item .\date.csv
- To apply your own data type to a custom object, you can use the PSTypeName property:
# You can even apply your own type name to your custom object
$jhp = [PSCustomObject]@{
PSTypeName = 'de.janhendrikpeters.awesomeclass'
TwitterHandle = 'NyanHP'
Born = [datetime]'1987-01-24'
}
$jhp | Get-Member
$jhp.GetType().FullName
# This method still knows the gory details
- PowerShell also allows you to add your own type name nearly everywhere:
# You can even add your own type to anything
$item = Get-Item -Path /
$item.PSTypeNames.Insert(0,'JHP')
$item | Get-Member