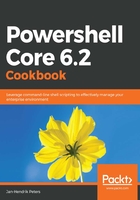
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- For this recipe, let's start with the humble FileInfo object and its members:
# To get started, we need something to manipulate
$tempFile = Get-Item -Path $(New-TemporaryFile).FullName
# The ubiquitous Get-Member shows all relevant details - for now
$tempFile | Get-Member
- Even with the Force parameter, there are no additional details that might help:
# Using the force does not provide more useful output
$tempFile | Get-Member -Force
- The GetType method, however, returns some useful methods that we can leverage:
# With reflection, we can dive deep into our objects
$tempFile.GetType() | Get-Member -Name Get*
- Try retrieving all the fields from your directory. Fields are usually private properties that can neither be read nor written to directly:
$tempFile.GetType().GetFields([Reflection.BindingFlags]::NonPublic -bor [Reflection.BindingFlags]::Instance) |
Format-Table -Property FieldType, Name
# To see the value of a field, try using the GetField method
$field = $tempFile.GetType().GetField('_name', [Reflection.BindingFlags]::NonPublic -bor [Reflection.BindingFlags]::Instance)
$field.GetValue($tempFile)
- Let's have some fun and modify the full name of a file:
$fullName = $tempFile.GetType().GetField('FullPath', [Reflection.BindingFlags]::NonPublic -bor [Reflection.BindingFlags]::Instance)
$fullName.GetValue($tempFile)
$fullName.SetValue($tempFile, 'C:\Users\japete.EUROPE\AppData\Local\Temp\WHATISHAPPENING')
- Observing the output, it doesn't look that bad:
$tempFile.FullName # Oh boy...
$tempFile # File still looks like before
$tempFile | Get-Member # Get-Member also looks normal
- However, object methods now fall apart:
$tempFile.CopyTo('D:\test')
Exception calling "CopyTo" with "1" argument(s): "Could not find file 'C:\Users\japete.EUROPE\AppData\Local\Temp\WHATISHAPPENING'."
At line:1 char:1
+ $tempFile.CopyTo('D:\test')
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : NotSpecified: (:) [], MethodInvocationException
+ FullyQualifiedErrorId : FileNotFoundException
- Using Add-Member is a bit less disruptive and still very useful. Try adding a note property and observe the output:
# Note properties are like yellow sticky notes - they are loosely attached to the object
$tempFile | Add-Member -NotePropertyName MyStickyNote -NotePropertyValue 'SomeValue'
$tempFile.MyStickyNote
# Note properties have a changeable data type
$tempFile.MyStickyNote = Get-Date
- A script property is a self-calculating property that might be useful as well:
# ScriptProperties are dynamic properties that calculate themselves.
# By using this, we are referencing the current instance of the class
$tempFile | Add-Member -MemberType ScriptProperty -Name Hash -Value {Get-FileHash -Path $this.PSPath}
$tempfile.Hash
- Lastly, a script method allows you to define something similar to an object method, with a script block as its payload:
# ScriptMethods are similar to object methods and can accept parameters as well
$tempFile | Add-Member -MemberType ScriptMethod -Name GetFileHash -Value {Get-FileHash -Path $this.PSPath}
$tempFile.GetFileHash()