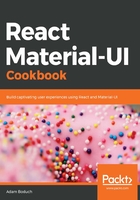
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have four Paper components to render in a grid. Inside each of these Paper components, you have three Chip components, which are nested grid items.
Here's what the code looks like:
import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import Paper from '@material-ui/core/Paper';
import Grid from '@material-ui/core/Grid';
import Chip from '@material-ui/core/Chip';
const styles = theme => ({
root: {
flexGrow: 1
},
paper: {
padding: theme.spacing(2),
textAlign: 'center',
color: theme.palette.text.secondary
}
});
const FillingSpace = withStyles(styles)(({ classes, justify }) => (
<div className={classes.root}>
<Grid container spacing={4}>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>
<Grid container justify={justify}>
<Grid item>
<Chip label="xs=12" />
</Grid>
<Grid item>
<Chip label="sm=6" />
</Grid>
<Grid item>
<Chip label="md=3" />
</Grid>
</Grid>
</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>
<Grid container justify={justify}>
<Grid item>
<Chip label="xs=12" />
</Grid>
<Grid item>
<Chip label="sm=6" />
</Grid>
<Grid item>
<Chip label="md=3" />
</Grid>
</Grid>
</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>
<Grid container justify={justify}>
<Grid item>
<Chip label="xs=12" />
</Grid>
<Grid item>
<Chip label="sm=6" />
</Grid>
<Grid item>
<Chip label="md=3" />
</Grid>
</Grid>
</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>
<Grid container justify={justify}>
<Grid item>
<Chip label="xs=12" />
</Grid>
<Grid item>
<Chip label="sm=6" />
</Grid>
<Grid item>
<Chip label="md=3" />
</Grid>
</Grid>
</Paper>
</Grid>
</Grid>
</div>
));
export default FillingSpace;
The justify property is specified on container Grid components. In this example, it's the container that contains the Chip components as items. Each container is using the flex-start value, which will align the Grid items at the start of the container. The result is as follows:
