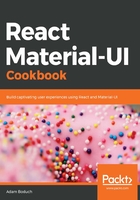
How it works...
Let's take a look at the state method and the onScroll() method of the ScrolledAppBar component:
state = {
scrolling: false,
scrollTop: 0
};
onScroll = e => {
this.setState(state => ({
scrollTop: e.target.documentElement.scrollTop,
scrolling:
e.target.documentElement.scrollTop > state.scrollTop
}));
};
componentDidMount() {
window.addEventListener('scroll', this.onScroll);
}
componentWillUnmount() {
window.removeEventListener('scroll', this.onScroll);
}
When the component mounts, the onScroll() method is added as a listener to the scroll event on the window object. The scrolling state is a Boolean value that hides the AppBar component when true. The scrollTop state is the position of the previous scroll event. The onScroll() method figures out whether the user is scrolling by checking if the new scroll position is greater than the last scroll position.
Next, let's take a look at the Fade component that's used to hide the AppBar component when scrolling, as follows:
<Fade in={!this.state.scrolling}>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
>
<MenuIcon />
</IconButton>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
My Title
</Typography>
<Button color="inherit">Login</Button>
</Toolbar>
</AppBar>
</Fade>
The in property tells the Fade component to fade its children, in, when the value is true. In this example, the condition is true when the scrolling state is false.