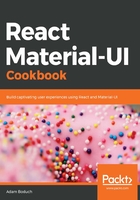
How it works...
Let's start by looking at the render() method of the MyToolbar component, as follows:
render() {
const { classes, title, MenuItems, RightButton } = this.props;
return (
<Fragment>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
onClick={e =>
this.setState({ anchor: e.currentTarget })
}
>
<MenuIcon />
</IconButton>
<Menu
anchorEl={this.state.anchor}
open={Boolean(this.state.anchor)}
onClose={this.closeMenu}
>
<MenuItems closeMenu={this.closeMenu} />
</Menu>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
{title}
</Typography>
<RightButton />
</Toolbar>
</AppBar>
<div className={classes.toolbarMargin} />
</Fragment>
);
}
This is where the AppBar component and the Toolbar components from Material-UI are rendered. A Fragment component is used because two elements are returned: the AppBar component and the <div> element that sets the top margin for the page content. Within the toolbar, you have the following:
- The menu button that displays the menu when clicked
- The menu itself
- The title
- The right-side button
From the MyToolbar properties, there are two components that render() uses: MenuItems and RightButton. In addition to the title prop, these are the parts of the AppBar component that you want to customize. The approach here is to define default values for these properties so that the AppBar component can be rendered:
static defaultProps = {
MenuItems: ({ closeMenu }) => (
<Fragment>
<MenuItem onClick={closeMenu}>Profile</MenuItem>
<MenuItem onClick={closeMenu}>My account</MenuItem>
<MenuItem onClick={closeMenu}>Logout</MenuItem>
</Fragment>
),
RightButton: () => <Button color="inherit">Login</Button>
};
You can pass custom values to these properties when you render MyToolbar. The defaults used here could be the values used for the home screen, for example.