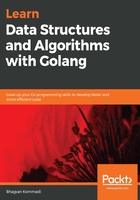
Tuples
A tuple is a finite sorted list of elements. It is a data structure that groups data. Tuples are typically immutable sequential collections. The element has related fields of different datatypes. The only way to modify a tuple is to change the fields. Operators such as + and * can be applied to tuples. A database record is referred to as a tuple. In the following example, power series of integers are calculated and the square and cube of the integer is returned as a tuple:
//main package has examples shown
// in Hands-On Data Structures and algorithms with Go book
package main
// importing fmt package
import (
"fmt"
)
//gets the power series of integer a and returns tuple of square of a
// and cube of a
func powerSeries(a int) (int,int) {
return a*a, a*a*a
}
The main method calls the powerSeries method with 3 as a parameter. The square and cube values are returned from the method:
// main method
func main() {
var square int
var cube int
square, cube = powerSeries(3)
fmt.Println("Square ", square, "Cube", cube)
}
Run the following commands:
go run tuples.go
The following screenshot displays the output:

The tuples can be named in the powerSeries function, as shown in the following code:
func powerSeries(a int) (square int, cube int) {
square = a*a
cube = square*a
return
}
If there is an error, it can be passed with tuples, as shown in the following code:
func powerSeries(a int) (int, int, error) {
square = a*a
cube = square*a
return square,cube,nil
}