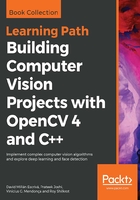
Creating the graphical user interface
Before we start with the image processing algorithms, we create the main user interface for our application. We are going to use the Qt-based user interface to allow us to create single buttons. The application receives one input parameter to load the image to process, and we are going to create four buttons, as follows:
- Show histogram
- Equalize histogram
- Lomography effect
- Cartoonize effect
We can see the four results in the following screenshot:

Let's begin developing our project. First of all, we are going to include the OpenCV – required headers, define an image matrix to store the input image, and create a constant string to use the new command-line parser already available from OpenCV 3.0; in this constant, we allow only two input parameters, help and the required image input:
// OpenCV includes #include "opencv2/core/utility.hpp" #include "opencv2/imgproc.hpp" #include "opencv2/highgui.hpp" using namespace cv; // OpenCV command line parser functions // Keys accepted by command line parser const char* keys = { "{help h usage ? | | print this message}" "{@image | | Image to process}" };
The main function starts with the command-line parser variable; next, we set the about instruction and print the help message. This line sets up the help instructions of our final executable:
int main(int argc, const char** argv) { CommandLineParser parser(argc, argv, keys); parser.about("Chapter 4. PhotoTool v1.0.0"); //If requires help show if (parser.has("help")) { parser.printMessage(); return 0; }
If the user doesn't require help, then we have to get the file path image in the imgFile variable string and check that all required parameters are added with the parser.check() function:
String imgFile= parser.get<String>(0); // Check if params are correctly parsed in his variables if (!parser.check()) { parser.printErrors(); return 0; }
Now, we can read the image file with the imread function, and then create the window in which the input image will be shown later with the namedWindow function:
// Load image to process Mat img= imread(imgFile); // Create window namedWindow("Input");
With the image loaded and the window created, we only need to create the buttons for our interface and link them with the callback functions; each callback function is defined in the source code and we are going to explain these functions later in this chapter. We are going to create the buttons with the createButton function with the QT_PUSH_BUTTON constant to button style:
// Create UI buttons createButton("Show histogram", showHistoCallback, NULL, QT_PUSH_BUTTON, 0); createButton("Equalize histogram", equalizeCallback, NULL, QT_PUSH_BUTTON, 0); createButton("Lomography effect", lomoCallback, NULL, QT_PUSH_BUTTON, 0); createButton("Cartoonize effect", cartoonCallback, NULL, QT_PUSH_BUTTON, 0);
To finish our main function, we show the input image and wait for a key press to finish our application:
// Show image imshow("Input", img); waitKey(0); return 0;
Now, we only have to define each callback function, and in the next sections, we are going to do just that.