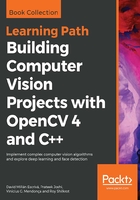
Image color equalization
In this section, we are going to learn how to equalize a color image. Image equalization, or histogram equalization, tries to obtain a histogram with a uniform distribution of values. The result of equalization is an increase in the contrast of an image. Equalization allows lower local contrast areas to gain high contrast, spreading out the most frequent intensities. This method is very useful when the image is extremely dark or bright and there is a very small difference between the background and foreground. Using histogram equalization, we increase the contrast and the details that are over- or under-exposed. This technique is very useful in medical images, such as X-rays.
However, there are two main disadvantages to this method: the increase in background noise and a consequent decrease in useful signals. We can see the effect of equalization in the following photograph, and the histogram changes and spreads when increasing the image contrast:

Let's implement our equalization histogram; we are going to implement it in the Callback function defined in the user interface's code:
void equalizeCallback(int state, void* userData)
{ Mat result; // Convert BGR image to YCbCr Mat ycrcb; cvtColor(img, ycrcb, COLOR_BGR2YCrCb); // Split image into channels vector<Mat> channels; split(ycrcb, channels); // Equalize the Y channel only equalizeHist(channels[0], channels[0]); // Merge the result channels merge(channels, ycrcb); // Convert color ycrcb to BGR cvtColor(ycrcb, result, COLOR_YCrCb2BGR); // Show image imshow("Equalized", result); }
To equalize a color image, we only have to equalize the luminance channel. We can do this with each color channel but the result is not usable. Alternatively, we can use any other color image format, such as HSV or YCrCb, that separates the luminance component in an individual channel. Thus, we choose YCrCb and use the Y channel (luminance) to equalize. Then, we follow these steps:
1. Convert or input the BGR image into YCrCb using the cvtColor function:
Mat result; // Convert BGR image to YCbCr Mat ycrcb; cvtColor(img, ycrcb, COLOR_BGR2YCrCb);
2. Split the YCrCb image into different channels matrix:
// Split image into channels vector<Mat> channels; split(ycrcb, channels);
3. Equalize the histogram only in the Y channel, using the equalizeHist function which has only two parameters, the input and output matrices:
// Equalize the Y channel only equalizeHist(channels[0], channels[0]);
4. Merge the resulting channels and convert them into the BGR format to show the user the result:
// Merge the result channels merge(channels, ycrcb); // Convert color ycrcb to BGR cvtColor(ycrcb, result, COLOR_YCrCb2BGR); // Show image imshow("Equalized", result);
The process applied to a low-contrast Lena image will have the following result:
