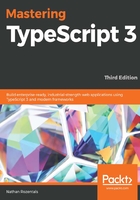
Tuples and rest syntax
Tuples can also use rest syntax, both in function definitions and in object rest and spread. As an example of using tuples and rest in a function definition, consider the following code:
function useTupleAsRest(...args: [number, string, boolean]) { let [arg1, arg2, arg3] = args; console.log(`arg1: ${arg1}`); console.log(`arg2: ${arg2}`); console.log(`arg3: ${arg3}`); } useTupleAsRest(1, "stringValue", false);
Here, we have defined a function named useTupleAsRest, which is using rest syntax, but also defining the args parameter as a tuple. This allows us to destructure the tuple into three variables named arg1, arg2, and arg3 on the first line of this function. We then log all three values to the console. When we call this function, we must provide three values that match the tuple types. The output of this code is as follows:
arg1: 1 arg2: stringValue arg3: false
Note that because the rest parameter is now a tuple, we will get compile errors if we attempt to call this function with anything other than a number, string, or boolean combination.
The second manner in which we can use rest syntax with tuples is in their definition. Consider the following code:
type RestTupleType = [number, ...string[]]; let restTuple: RestTupleType = [1, "string1", "string2", "string3"];
Here, we have defined a type named RestTupleType, which is a tuple with the first property of a number, and then a variable number of strings (using rest syntax). We are then creating a variable named restTuple with the number 1, and the strings "string1", "string2", and "string3" as properties.