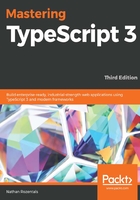
Class constructors
Classes can accept parameters during their initial construction. This allows us to combine the creation of a class and the setting of its parameters into a single line of code. Consider the following class definition:
class ClassWithConstructor { id: number; name: string; constructor(_id: number, _name: string) { this.id = _id; this.name = _name; } }
Here, we have defined a class named ClassWithConstructor that has two properties, an id property of type number, and a name property of type string. It also has a constructor function that accepts two parameters. The constructor function is assigning the value of the _id argument to the class property of id, and the value of the _name argument to the class property, name. Note that, because we are initializing both the id and name properties within the class constructor, we know that any class created will have set a value for these properties. We therefore do not need to use the unspecified type in this instance.
We can then construct an instance of this class as follows:
var classWithConstructor = new ClassWithConstructor(1, "name"); console.log(`classWithConstructor =
${JSON.stringify(classWithConstructor)}`);
The first line of this code creates an instance of the ClassWithConstructor class, using the constructor function. We are then simply logging a JSON version of this class to the console. The output of this code is as follows:
classWithConstructor = {"id":1,"name":"name"}