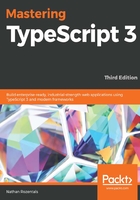
Class modifiers
As we discussed briefly in the opening chapter, TypeScript introduces the public and private access modifiers to mark class variables and functions as either public or private. Additionally, we can also use the protected access modifier, which we will discuss a little later.
A public class property can be accessed by any calling code. Consider the following code:
class ClassWithPublicProperty { public id: number | undefined; } let publicAccess = new ClassWithPublicProperty(); publicAccess.id = 10;
Here, we have defined a class named ClassWithPublicProperty that has a single property named id. We then create an instance of this class, named publicAccess, and assign the value of 10 to the id property of this instance. Let's now explore how marking a property private will affect access to this property, as follows:
class ClassWithPrivateProperty { private id: number; constructor(_id : number) { this.id = _id; } } let privateAccess = new ClassWithPrivateProperty(10); privateAccess.id = 20;
Here, we have defined a class named ClassWithPrivateProperty that has a single property named id, and which has now been marked as private. This class also has a constructor function that takes a single argument, named _id, and assigns the value of this argument to the id property. Note that we are using the this.id syntax in this assignment.
We then create an instance of this class, named privateAccess, and then attempt to assign the value 20 to the private id property. This code, however, will generate the following error:
error TS2341: Property 'id' is private and only accessible within class 'ClassWithPrivateProperty'.
As we can see from the error message, TypeScript will not allow assignment to the id property of this class outside the class itself, as we have marked it as private. Note that we are able to assign a value to the id property from within the class, as we have done in the constructor function.