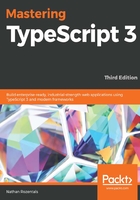
Function overriding
The constructor of a class is, however, just a function. In the same way that we can use the super keyword in a constructor, we can also use the super keyword when a base class and its derived class use the same function name. This technique is called function overriding. In other words, the derived class has a function name that is the same name as that of a base class function, and it overrides this function definition. Consider the following code:
class BaseClassWithFunction { public id : number | undefined; getProperties() : string { return `id: ${this.id}`; } } class DerivedClassWithFunction extends BaseClassWithFunction { public name: string | undefined; getProperties() : string { return `${super.getProperties()}` + ` , name: ${this.name}`; } }
Here, we have defined a class named BaseClassWithFunction that has a public id property, and a function named getProperties, which just returns a string representation of the properties of the class. Our DerivedClassWithFunction class, however, also includes a function called getProperties. This function is a function override of the getProperties base class function. In order to call through to the base class function, we need to use the super keyword, as shown in the call to super.getProperties.
Let's take a look at how we would use these classes:
var derivedClassWithFunction = new DerivedClassWithFunction(); derivedClassWithFunction.id = 1; derivedClassWithFunction.name = "derivedName"; console.log(derivedClassWithFunction.getProperties());
This code creates a variable named derivedClassWithFunction, which is an instance of the DerivedClassWithFunction class. It then sets both the id and name properties, and then logs the result of calling the getProperties function to the console. This code snippet will result in the following:
id: 1 , name: derivedName
The results show that the getProperties function of the derivedClassWithFunction variable will call through to the base class getProperties function, as expected.