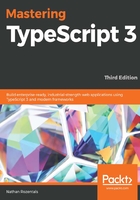
instanceof
TypeScript also allows us to check whether an object has been created from a specific class using the instanceof keyword. To illustrate this, consider the following set of classes:
class A { } class BfromA extends A { } class CfromA extends A { } class DfromC extends CfromA { }
Here, we have defined four classes, starting with a simple class named A. We then define two classes derived from A, called BfromA and CfromA. The final class definition is for a class named DfromC, which is derived from class CfromA. Let's now use the instanceof keyword to find out what the compiler thinks of this inheritance structure, as follows:
function checkInstanceOf(value: A | BfromA | CfromA | DfromC) { console.log(`checking instanceof :`) if (value instanceof A) { console.log(`found instanceof A`); } if (value instanceof BfromA) { console.log(`found instanceof BfromA`); } if (value instanceof CfromA) { console.log(`found instanceof CFromA`); } if (value instanceof DfromC) { console.log(`found instanceof DfromC`); } }
Here, we have created a function named checkInstanceOf that has a single parameter, which can be any one of our four class types. Once we are inside the function, we log a message to the console indicating that we are starting to check the input argument. We then have four if statements, each of which is checking to see whether the type of the value argument matches any one of our four class types.
Let's use this function as follows:
checkInstanceOf(new A()); checkInstanceOf(new BfromA()); checkInstanceOf(new CfromA()); checkInstanceOf(new DfromC());
Here, we are calling the checkInstanceOf function with an instance of each of our four classes. The output of this code is as follows:

Here, we can see that, if we call this function with a class of type A, our code is detecting this correctly, since if (value instanceof A) is returning true. Interestingly, when we send an instance of the BfromA class, we can see that both the instanceof A and the instanceof BfromA statements are returning true. This means that the compiler is correctly detecting that the BfromA class has been derived from A. The same logic applies to the class CfromA. Observe how the DfromC class will cause three of the instanceof operators to return true. Our code is detecting that DfromC is derived from CfromA, and that because CfromA derives from A, DfromC is also an instance of type A.