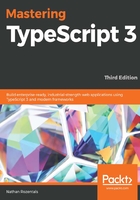
The Person class
To simplify our code, we will create an abstract base class to hold all code that is common to infants, children, and adults. Again, abstract classes can never be instantiated, and, as such, are designed to be derived from. We will call this class Person, as follows:
abstract class Person implements IPerson { Category: PersonCategory; private DateOfBirth: Date; constructor(dateOfBirth: Date) { this.DateOfBirth = dateOfBirth; this.Category = PersonCategory.Undefined; } abstract canSignContracts(): boolean printDetails() : void { console.log(`Person : `); console.log(`Date of Birth : ` + `${this.DateOfBirth.toDateString()}`); console.log(`Category : ` + `${PersonCategory[this.Category]}`); console.log(`Can sign : ` + `${this.canSignContracts()}`); } }
This abstract Person class implements the IPerson interface, and as such, will need three things: a Category property, a canSignContracts function, and a printDetails function. In order to print the person's date of birth, we also need a DateOfBirth property, which we will set in our constructor function.
There are a couple of interesting things to note about this Person class. Firstly, the DateOfBirth property has been declared private. This means that the only class that has access to the DateOfBirth property is the Person class itself. Our requirements do not mention using the date of birth outside of the printing function, so there is no need to access or modify the date of birth once it has been set.
Secondly, the canSignContracts function has been marked as abstract. This means that any class that derives from this class is forced to implement the canSignContracts function, which is exactly what we wanted.
Thirdly, the printDetails function has been fully implemented in this abstract class. This means that a single print function is automatically available for any class that derives from Person.