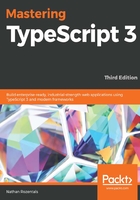
Using the Factory class
To illustrate how simple it becomes to use this PersonFactory class, consider the following code:
let factory = new PersonFactory(); let p1 = factory.getPerson(new Date(2017, 0, 20)); p1.printDetails(); let p2 = factory.getPerson(new Date(2010, 0, 20)); p2.printDetails(); let p3 = factory.getPerson(new Date(1969, 0, 20)); p3.printDetails();
We start with the creation of a variable, personFactory, to hold a new instance of the PersonFactory class. We then create three variables, named p1, p2, and p3, by calling the getPerson function of the PersonFactory, passing in three different dates to the same function. The output of this code is as follows:

We have satisfied our business requirements and implemented a very common design pattern at the same time. If we look back at our code, we can see that we have a few well-defined and simple classes. Our Infant, Child, and Adult classes are only concerned with logic relating to their classification, and whether they can sign contracts. Our Person abstract base class is only concerned with logic related to the IPerson interface, and PersonFactory is only concerned with the logic surrounding the date of birth. This sample illustrates how object-oriented design patterns and the object-oriented features of the TypeScript language can help with writing good, extensible, and maintainable code.