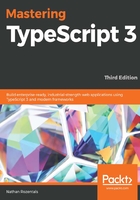
Decorator syntax
A decorator is simply a function that is called with a specific set of parameters. These parameters are automatically populated by the JavaScript runtime, and contain information about the class to which the decorator has been applied. The number of parameters and the types of these parameters determine where a decorator can be applied. To illustrate the syntax used for decorators, let's define a simple class decorator, as follows:
function simpleDecorator(constructor : Function) { console.log('simpleDecorator called.'); }
Here, we have defined a function named simpleDecorator that takes a single parameter, named constructor of type Function. This simpleDecorator function logs a message to the console indicating that it has been called. This function is our decorator definition. In order to use it, we will need to apply it to a class definition, as follows:
@simpleDecorator class ClassWithSimpleDecorator { }
Here, we have applied the decorator to the class definition of ClassWithSimpleDecorator by using the "at" symbol (@), followed by the decorator name. Running this simple decorator code will produce the following output:
simpleDecorator called.
Note, however, that we have not created an instance of the class as yet. We have simply specified the class definition, added a decorator to it, and our decorator function has automatically been called. This indicates to us that the decorators are applied when a class is being defined, and not when it is being instantiated. As a further example of this, consider the following code:
let instance_1 = new ClassWithSimpleDecorator(); let instance_2 = new ClassWithSimpleDecorator(); console.log(`instance_1 : ${JSON.stringify(instance_1)}`); console.log(`instance_2 : ${JSON.stringify(instance_2)}`);
Here, we have created two instances of the ClassWithSimpleDecorator class, named instance_1 and instance_2. We are then simply logging a message to the console. The output of this code snippet is as follows:
simpleDecorator called. instance_1 : {} instance_2 : {}
What this output shows us is that the decorator function has only been called once, no matter how many instances of the same class have been created or used. Decorators are only invoked as the class is being defined.