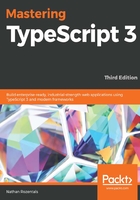
Union types
TypeScript allows us to express a type as the combination of two or more other types. These types are known as union types, and their declaration uses the pipe symbol (|) to list all of the types that will make up the new type. Consider the following TypeScript code:
var unionType : string | number; unionType = 1; console.log(`unionType : ${unionType}`); unionType = "test"; console.log(`unionType : ${unionType}`);
Here, we have defined a variable named unionType, which uses the union type syntax to denote that it can hold either a string or a number. We are then assigning a number to this variable, and logging its value to the console. We then assign a string to this variable, and again log its value to the console. This code snippet will output the following:
unionType : 1 unionType : test
Using union types may seem a little strange at first. After all, why would a simple variable need to hold both a number and a string? Surely this is breaking our strong typing rules in some way. The benefits of union types, however, can be seen when using functions, or when calling REST endpoints that may return different response structures.