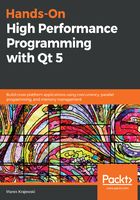
Using Qt Creator's heob integration
Sometimes, the previous analysis isn't enough, as it will only show that our program accumulates memory, but cannot say if it is doing that by design (building up a great cache?), or because we have a memory leak. In such cases, we will have to check whether memory is leaking—a very arduous task that is best left to specialized tools.
For that reason Qt Creator supports Valgrind's Memecheck application on Linux, which unfortunately isn't available on Windows. Admittedly, in the case of memory profiling, the situation isn't quite as dim as with CPU profiling, because Qt Creator has built-in integration for the heob memory-checking tool.
However, heob isn't contained in Qt Creator's installation and has to be installed manually. Go to https://github.com/ssbssa/heob/releases and download the latest compressed archive (heob-2.1.7z in my case). It contains heob's two binaries, for 32-bits and 64-bits, decompress it and save it in your tools folder. As we are using MinGW, we will additionally need the dwarfstack DLLs to be able to read program symbols information. Go to https://github.com/ssbssa/dwarfstack/releases, download the latest compressed archive (dwarfstack-2.0-dlls.7z in my case), and decompress it in the same directory you saved the heob executables in.
To test leak detection, we will add the following two lines to the main.cc file of the example project, exactly as we did in the CPU case:
// induce memory leak
makeLeak();
return app.exec();
The makeLeak() function will allocate 10,000 bytes and simply leak it. Now click Analyze | Heob, and the following dialog will appear:

After you have fixed the problem with a workaround, open the Heob dialog, and enter the path where you stored the heob executables in the Heob path field, accept all the default settings, and click on OK. As seen in the next screenshot, heob will start the tested executable and inject its instrumented memory management functions:

After we stop the tested application, the detected memory problems will be reported in the Memcheck output panel, as seen in the following screenshot:

We see that our self-inflicted memory leak got detected. When we click on the link with the source location, the referenced line will be shown in the editor. Additionally, a tooltip containing information about the detected leak will be displayed when we hover the mouse above a given line.
Heob can do more than that, though; for example, it can check buffer overflows and invoke an exception or start a debugger when they occur. Qt Creator Manual provides documentation for the options shown in the dialog (see https://doc.qt.io/qtcreator/creator-heob.html), but there are more options than that. You can get a quick overview of the supported options when you invoke heob with the -H option, either from the dialog or directly.