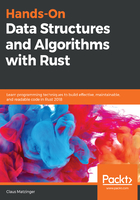
Objects and behavior
Organizing code in Rust is a bit different from regular object-oriented languages such as C#. There, an object is supposed to change its own state, interfaces are simple contract definitions, and specialization is often modeled using class inheritance:
class Door {
private bool is_open = false;
public void Open() {
this.is_open = true;
}
}
With Rust, this pattern would require constant mutability of any Door instance (thereby requiring explicit locking for thread safety), and without inheritance GlassDoor would have to duplicate code, making it harder to maintain.
Instead, it's recommended to create traits to implement (shared) behavior. Traits have a lot in common with abstract classes in traditional languages (such as default implementations of methods/functions), yet any struct in Rust can (and should) implement several of those traits:
struct Door {
is_open: bool
}
impl Door {
fn new(is_open: bool) -> Door {
Door { is_open: is_open }
}
}
trait Openable {
fn open(&mut self);
}
impl Openable for Door {
fn open(&mut self) {
self.is_open = true;
}
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn open_door() {
let mut door = Door::new(false);
door.open();
assert!(door.is_open);
}
}
This pattern is very common in the standard library, and often third-party libraries will even add behavior to existing types by implementing traits in their code (also known as extension traits).
Other than a typical class, where data fields and methods are in a single construct, Rust emphasizes the separation between those by declaring a struct for data and an impl part for the methods/functions. Traits name and encapsulate behaviors so they can easily be imported, shared, and reused.