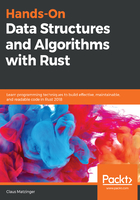
Exceptional lifetimes
Some lifetimes are different and Rust denominates them with a '. While this could be the predefined 'static, it's equally possible to create your own, something that is often required when working with structures.
This makes sense when thinking about the underlying memory structure: if an input parameter is passed into the function and returned at the end, its lifetime surpasses the function's. While the function owns this part of the memory during its lifetime, it cannot borrow a variable for longer than it actually exists. So, this snippet cannot work:
fn another_function(mut passing_through: MyStruct) -> MyStruct {
let x = vec![1, 2, 3];
// passing_through cannot hold a reference
// to a shorter lived x!
// the compiler will complain.
passing_through.x = &x;
return passing_through;
} // x's life ends here
The reason is that the passing_through variable outlives x. There are several solutions to this problem:
- Change the type definition of MyStruct to require ownership. This way, the structure now owns the variable and it will live as long as the structure:
fn another_function(mut passing_through: MyStruct) -> MyStruct {
let x = vec![1, 2, 3];
// passing_through owns x and it will be
// dropped together with passing_through.
passing_through.x = x;
return passing_through;
}
- Clone x to pass ownership into passing_through:
fn another_function(mut passing_through: MyStruct) -> MyStruct {
let x = vec![1, 2, 3];
let y = &x;
// passing_through owns a deep copy of x'value that is be
// dropped together with passing_through.
passing_through.x = y.clone();
return passing_through;
}
- In this case, vec![] is statically defined, so it could make sense to add it as a function parameter. This is not only more allocation-efficient, but also can enforce an appropriate lifetime:
fn another_function<'a>(mut passing_through: MyStruct<'a>, x: &'a Vec<u32>) -> MyStruct<'a> {
// The compiler knows and expects a lifetime that is
// at least as long as the struct's
// of any reference passed in as x.
passing_through.x = x;
return passing_through;
}
Lifetimes cause a lot of strange errors for many Rust users, and in the 2018 edition there is one less to worry about. With the introduction of non-lexical lifetimes, the borrow checker got a lot smarter and it is now able to check—up to a certain degree—semantically whether the variable was used. Recall from the rules of borrowing that, if a mutable reference is created, no immutable references can exist.
This code did not compile before Rust 1.31:
fn main() {
let mut a = 42;
let b = &a; // borrow a
let c = &mut a; // borrow a again, mutably
// ... but don't ever use b
}
Now it will compile since the compiler does not just check the beginning and ending of a scope, but also if the reference was used at all.