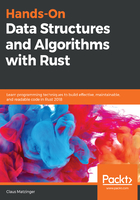
Log replay
Typically in databases, transaction logs are a resilience measure if something bad happens that the database must be restored—or to keep a replica up to date. The principle is fairly simple: the log represents a timeline of commands that have been executed in this exact order. Thus, to recreate that final state of a database, it is necessary to start with the oldest entry and apply every transaction that follows in that very order.
You may have caught how that fits the capabilities of a linked list nicely. So, what is missing from the current implementation?
The ability to remove elements starting at the front.
Since the entire data structure resembles a queue, this function is going to be called pop, as it's the typical name for this kind of operation. Additionally, pop will consume the item that was returned, making the list a single-use structure. This makes sense, to avoid replaying anything twice!
This looks a lot more complex than it is: the interior mutability pattern certainly adds complexity to the implementation. However, it makes the whole thing safe—thanks to RefCells checking borrowing rules at runtime. This also leads to the chain of functions in the last part—it retrieves the value from within its wrappers:
pub fn pop(&mut self) -> Option<String> {
self.head.take().map(|head| {
if let Some(next) = head.borrow_mut().next.take() {
self.head = Some(next);
} else {
self.tail.take();
}
self.length -= 1;
Rc::try_unwrap(head)
.ok()
.expect("Something is terribly wrong")
.into_inner()
.value
})
}
Calling this function in sequence returns the commands in the order they were inserted, providing a nice replay feature. For a real-world usage, it's important to provide the ability to serialize this state to disk as well, especially since this operation consumes the list entirely. Additionally, handling errors gracefully (instead of panicking and crashing) is recommended.