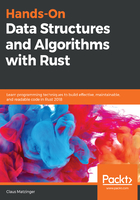
Examining the log
Looking at the list without consuming it is an iterator's job (see the info box), which—in Rust as well as in most other languages—is a simple implementation of an interface or trait. In fact, this is so common that the Rust docs have a great article (https://doc.rust-lang.org/std/iter/index.html#implementing-iterator), which is exactly what's required.
Since we are already working with heap references, the iterator can simply save an optional reference to a node and it's easy to move it forward and backward:
pub struct ListIterator {
current: Link,
}
impl ListIterator {
fn new(start_at: Link) -> ListIterator {
ListIterator {
current: start_at,
}
}
}
As the documentation states, a for loop uses two traits: Iterator and IntoIterator. Implementing the former is usually a good idea, as it provides access to the powerful methods in Iterator, such as map, fold, and so on, and nicely chains together with other—compatible—iterators:
impl Iterator for ListIterator {
type Item = String;
fn next(&mut self) -> Option<String> {
let current = &self.current;
let mut result = None;
self.current = match current {
Some(ref current) => {
let current = current.borrow();
result = Some(current.value.clone());
current.next.clone()
},
None => None
};
result
}
}
This iterator is responsible for moving one direction: forward. How can we walk back too?