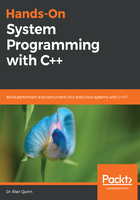
Scope
One addition to the C language that distinguishes it dramatically from assembly language programming is the use of scope. In assembly, a function prefix and postfix must be hand-coded, and the process for doing this depends entirely on the instruction set architecture (ISA) your CPU provides, and the ABI the programmer decides to use.
In C, the scope of a function is defined automatically for you using the {} syntax. For example:
#include <stdio.h>
int main(void)
{
printf("Hello World\n");
}
In our simple Hello World\n example, scope is used to define the start and end of our main() function. The scope of other primitives can also be defined using the {} syntax. For example:
#include <stdio.h>
int main(void)
{
int i;
for (i = 0; i < 10; i++) {
printf("Hello World: %d\n", i);
}
}
In the previous example, we define the scope of both our main() function and our for() loop.
The {} syntax can also be used to create scope for anything. For example:
#include <stdio.h>
int main(void)
{
{
int i;
...
}
{
int i;
...
}
}
In the previous example, we are able to use the i variable twice without accidentally redefining it, because we wrapped the definition of i in a {}. Not only does this tell the compiler the scope of i, it also tells the compiler to automatically create a prefix and postfix for us if they are needed (as optimizations can remove the need for a prefix and postfix).
Scope is also used to define what the compiler exposes with respect to linking. In standard C, the static keyword tells the compiler that a variable is only visible (that is, scoped) to the object file it is being compiled to, providing not only an optimization to the linker, but also preventing two global variables or functions from colliding with each other.
For this reason, if a function is not intended to be called by another source file (or library), it should be labeled static.
In the context of system programming, scope is important because system programming typically requires the acquisition of system-level resources. As will be seen in Chapter 4, C++, RAII, and the GSL Refresher, C++ provides the ability to create objects whose life can be scoped using standard C {} syntax, providing a safe mechanism for resource acquisition and release.